Android Jetpack Compose – Button
Android Jetpack Button composable is used to display a button UI element, and execute code when user clicks on it.
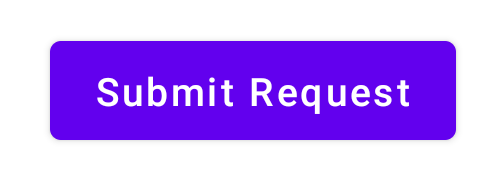
Example
In this example, we shall display a Button composable with text Submit Request
.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.layout.*
import androidx.compose.material.Button
import androidx.compose.material.Text
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxWidth()
.fillMaxHeight()
.padding(20.dp)) {
Button(onClick = { }) {
Text("Submit Request")
}
}
}
}
}
}
Screenshot
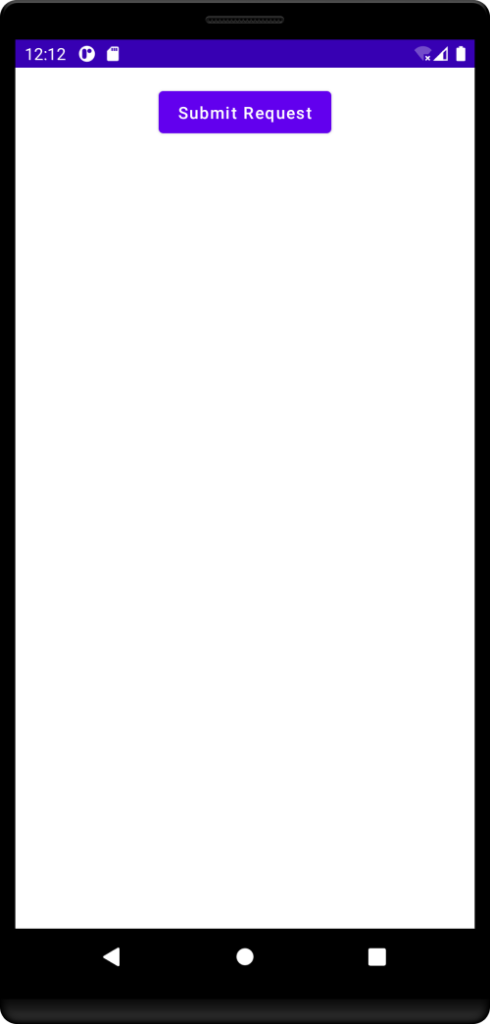
When we click on this button, nothing happens, because we have not written any code in it.
Let us display a Toast message when user clicks the button.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import android.widget.Toast
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.layout.*
import androidx.compose.material.Button
import androidx.compose.material.Text
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxWidth()
.fillMaxHeight()
.padding(20.dp)) {
Button(onClick = {
Toast.makeText(applicationContext, "You clicked the Button.", Toast.LENGTH_LONG).show()
}) {
Text("Submit Request")
}
}
}
}
}
}
Screenshot
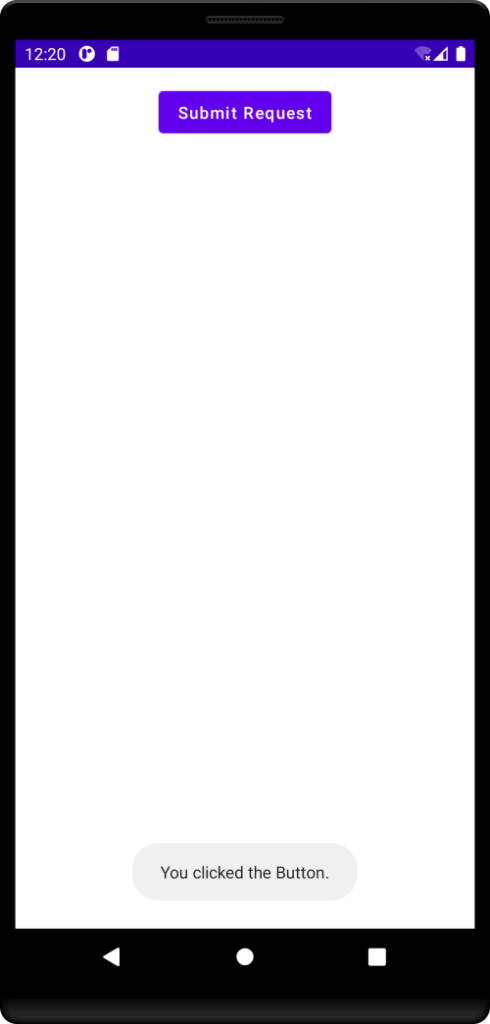
Instead of Toast, you may write your own code to execute when user clicks the button.
Conclusion
In this Android Jetpack Compose Tutorial, we learned what Button composable is, and how to style the Button composable, with the help of individual tutorials for each concept.