Android Compose – Set Circle Shape for Image
To set circular shape for Image, in Android Compose, wrap the image in Box composable and apply clip modifier with CircleShape.
The following code snippet shows how to set corner radius for Image with 10dp
.
Image(
painter = painterResource(id = R.drawable.mountains),
contentDescription = null,
modifier = Modifier.clip(CircleShape),
)
Example
In this example, let us display an Image with circular shape. We have taken an image with aspect ratio of 1:1, meaning width and height of the image are same.
If the width and height of the image are not equal, the Circle shape would look like as rounded corners.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
</>
Copy
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.shape.CircleShape
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier.fillMaxWidth(), content = {
Spacer(modifier = Modifier.height(10.dp))
Image(
painter = painterResource(id = R.drawable.mountains),
contentDescription = null,
modifier = Modifier.clip(CircleShape),
)
}
)
}
}
}
}
Screenshot
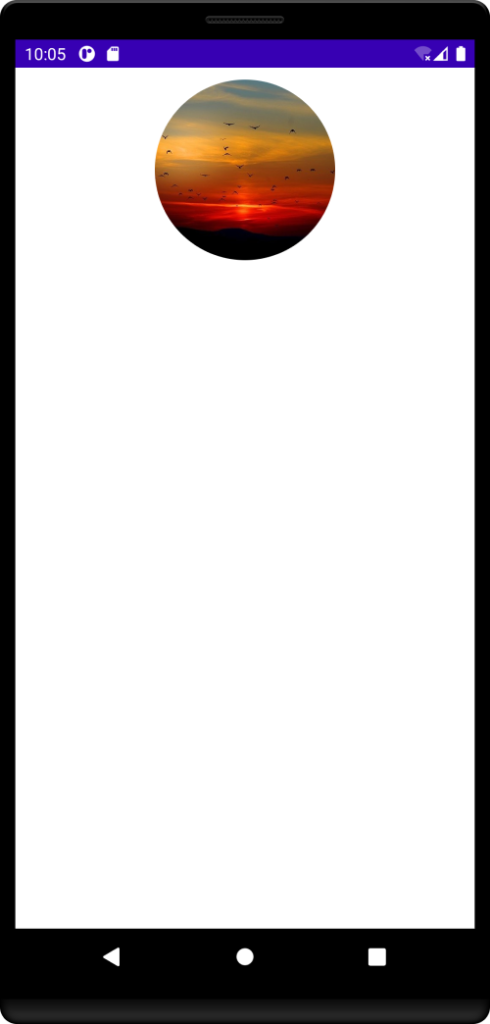
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to display Circle shaped Image.