Android Compose Row – Fill Maximum Width
To setup Row composable to fill maximum available width given to it from its parent, call fillMaxWidth
function on the Modifier object of this Row.
The following code snippet shows how to setup Row composable to fill maximum available width allowed by its parent.
Row( modifier = Modifier .fillMaxWidth() ) { ... }
Example
In this example, let us display two Row composables. The first Row composable has default width while the second Row composable is set to fill maximum width.
Inside each Row composable, we will have two Box composables. By default Row composable wraps around its content. So, for the first Row, the width is the result of its children. But the second Row should fill the maximum allowed width, irrespective of its children.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.background import androidx.compose.foundation.layout.* import androidx.compose.ui.Modifier import androidx.compose.ui.graphics.Color import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column { Row( modifier = Modifier .padding(10.dp) .background(Color.Gray), ) { Box(Modifier.size(100.dp).padding(10.dp).background(Color.Yellow)) Box(Modifier.size(100.dp).padding(10.dp).background(Color.Yellow)) } Row( modifier = Modifier .padding(10.dp) .fillMaxWidth() .background(Color.Gray), ) { Box(Modifier.size(100.dp).padding(10.dp).background(Color.Yellow)) Box(Modifier.size(100.dp).padding(10.dp).background(Color.Yellow)) } } } } } }
Screenshot
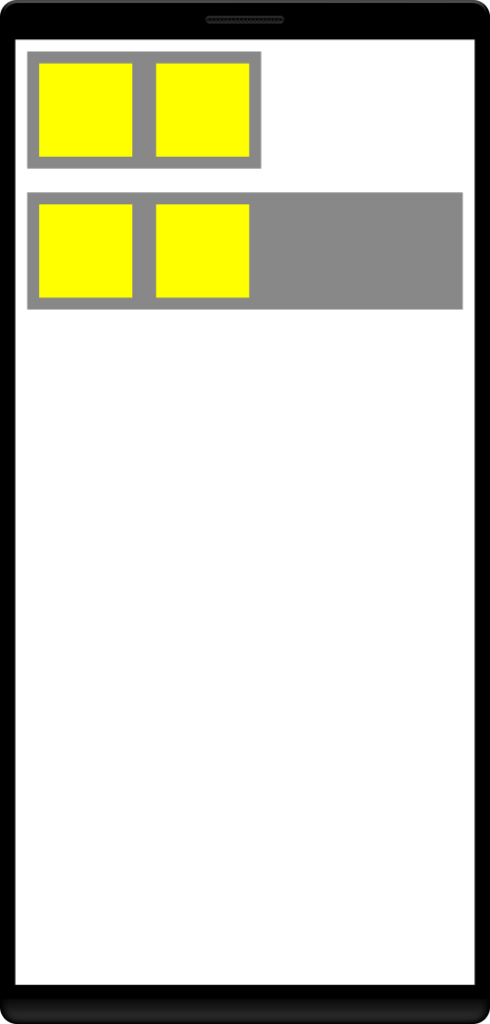
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to align contents of a Row composable to the center.