Android Compose – Button Background Color
In this tutorial, we will learn how to set a background color for a Button in Android Compose.
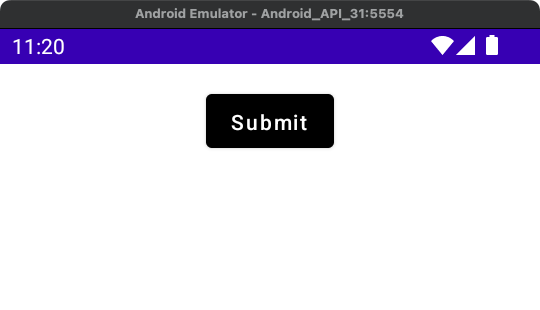
To set background color for a Button in Android Jetpack Compose, set colors parameter with the required ButtonColors value.
Button(colors = buttonDefaults.outlinedButtonColors(backgroundColor = Color.Black),)
buttonDefaults.outlinedButtonColors() returns ButtonColors object with given background color value.
Example
In this example, we have UI with a Button. The button has the text Submit
. And we set an yellow background color for this Button using colors parameter.
Create a Project in Android Studio with empty compose activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material.Button
import androidx.compose.material.ButtonDefaults
import androidx.compose.material.Text
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.platform.LocalContext
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.padding(20.dp)
.fillMaxWidth()) {
val context = LocalContext.current
Button(
colors = ButtonDefaults.outlinedButtonColors(backgroundColor = Color.Yellow),
onClick = {}
) {
Text("Submit")
}
}
}
}
}
}
Screenshot
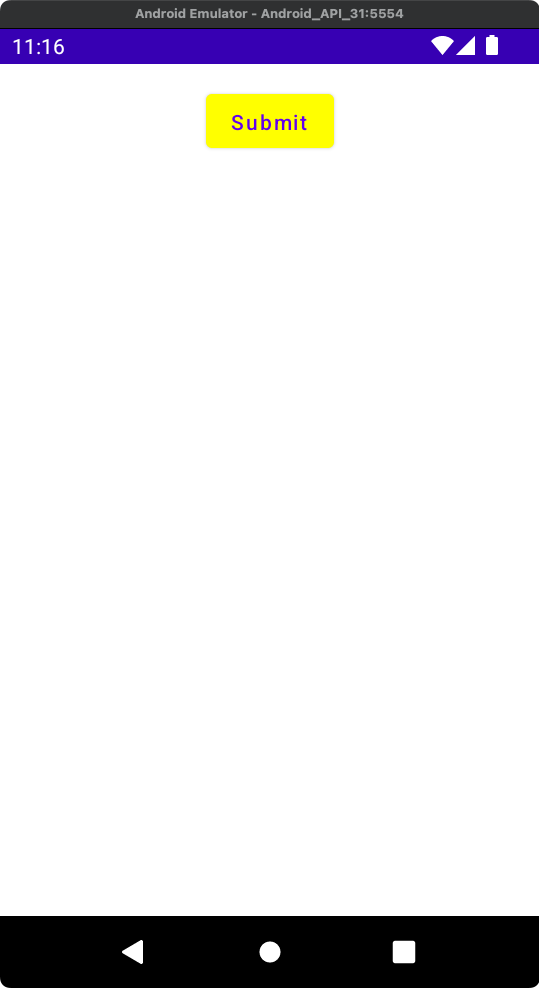
Compose Button with black background color
Now, let us change the background color of the button to black.
Code for Button
Button(
colors = ButtonDefaults.outlinedButtonColors(backgroundColor = Color.Black),
onClick = {},
) {
Text("Submit",
color = Color.White,
)
}
Screenshot
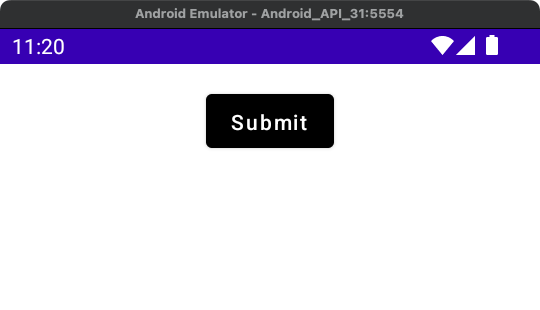
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set background color for a Button using colors parameter.