Android Compose – Blur Image
In this tutorial, we will learn how to blur an image displayed in Image composable in Android Compose.
Original Image
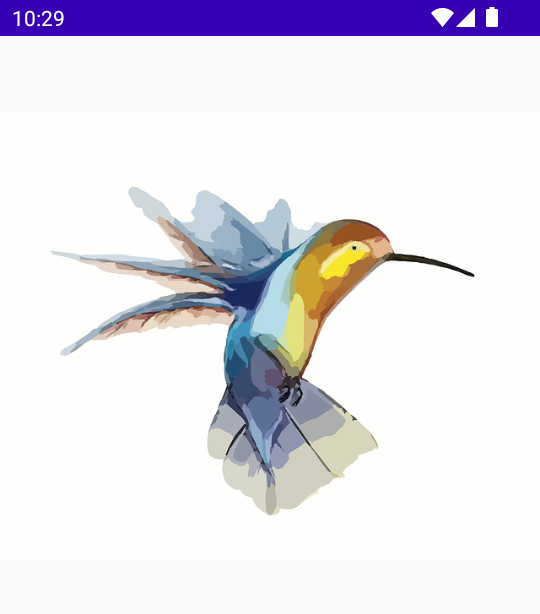
Blurred Image
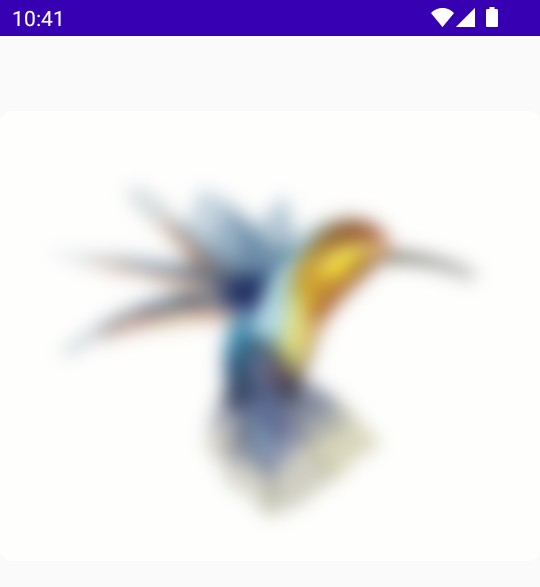
To blur an image in an Image composable in Android Jetpack Compose, use Modifier.blur() function. The blur() function takes the amount of blur along X-axis and Y-axis, and edge treatment as parameters.
Image(
painter = painterResource(id = R.drawable.hummingbird),
contentDescription = null,
modifier = Modifier
.blur(
radiusX = 10.dp,
radiusY = 10.dp,
edgeTreatment = BlurredEdgeTreatment(RoundedCornerShape(8.dp))
),
)
Example
In this example, we have Compose Activity with an Image composable. We blur the image by using Modifier.blur(). We set the blur radius to 10.dp
along X and Y axes.
Screenshot – Image with no blur
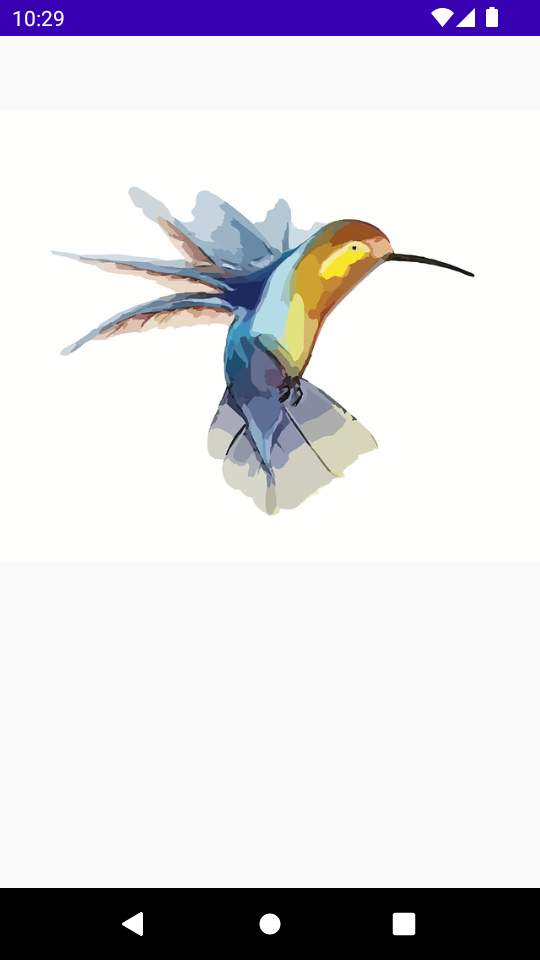
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.BlurredEdgeTreatment
import androidx.compose.ui.draw.blur
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier.fillMaxWidth(), content = {
Spacer(modifier = Modifier.height(50.dp))
Image(
painter = painterResource(id = R.drawable.hummingbird),
contentDescription = null,
modifier = Modifier
.blur(
radiusX = 10.dp,
radiusY = 10.dp,
edgeTreatment = BlurredEdgeTreatment(RoundedCornerShape(8.dp))
),
)
}
)
}
}
}
}
Screenshot – Image with blur
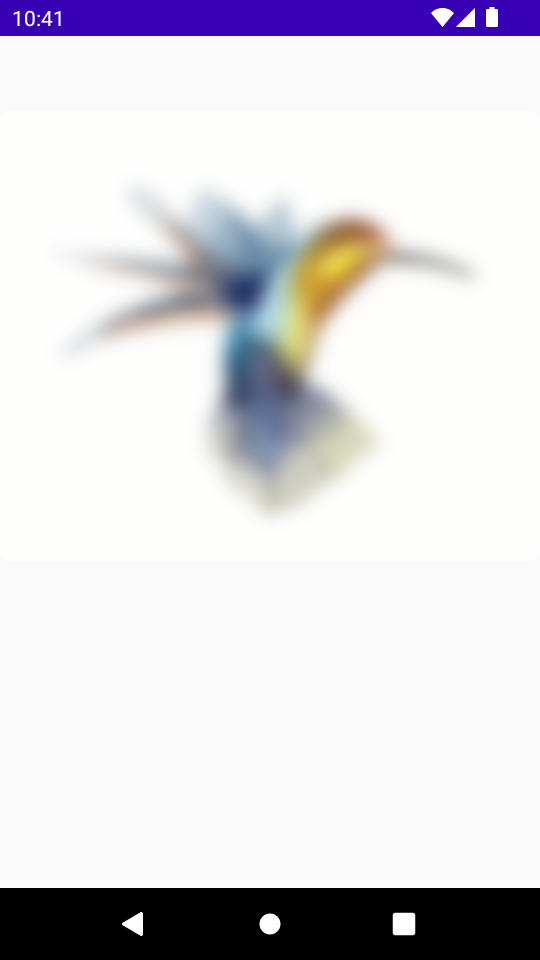
Project Download
The complete Android Studio Project in the above example is available for download at the following link. Download the ZIP file, uncompress it, and open with Android Studio.
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to blur the image in an Image composable by using Modifier.blur() function.