Android Compose – Button Padding
In this tutorial, we will learn how to set content padding for a Button in Android Compose.
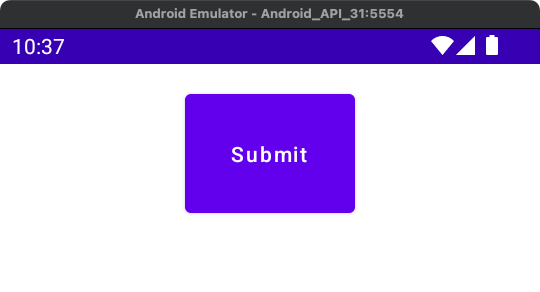
To set content padding for the Button in Android Jetpack Compose, set contentPadding parameter with the required PaddingValues.
Button(contentPadding = PaddingValues(30.dp),)
Example
In this example, we have UI with a Button. The button has the text Submit
. And we set padding for this Button to 30.dp
on all sides.
Create a Project in Android Studio with empty compose activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import androidx.compose.foundation.layout.* import androidx.compose.material.Button import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.platform.LocalContext import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier .padding(20.dp) .fillMaxWidth()) { val context = LocalContext.current Button( contentPadding = PaddingValues(30.dp), onClick = {} ) { Text("Submit") } } } } } }
Screenshot
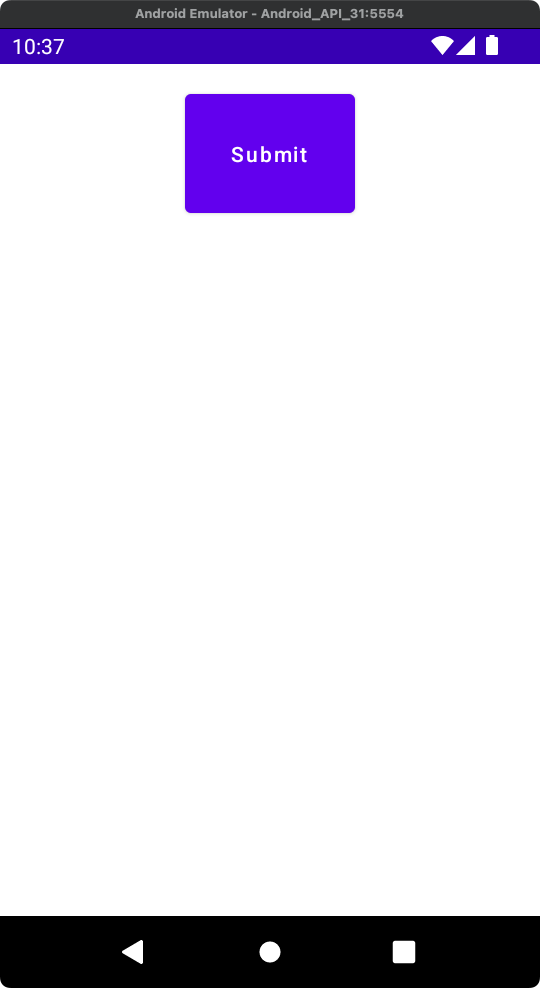
ADVERTISEMENT
Padding of 10.dp on all sides
Now, let us change the padding to 10.dp
on all sides.
Button( contentPadding = PaddingValues(10.dp), onClick = {} ) { Text("Submit") }
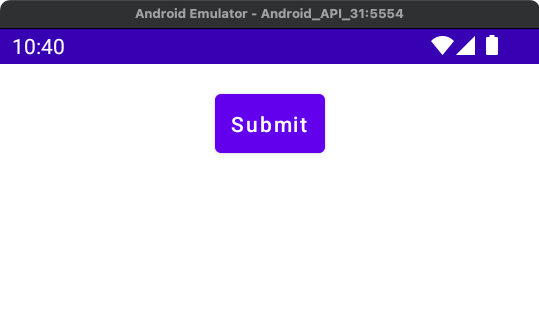
Padding of 10.dp on all left and right, and 5.dp on top and bottom
Now, let us change the padding to 10.dp
on all sides.
Button( contentPadding = PaddingValues(horizontal = 50.dp, vertical = 5.dp), onClick = {} ) { Text("Submit") }
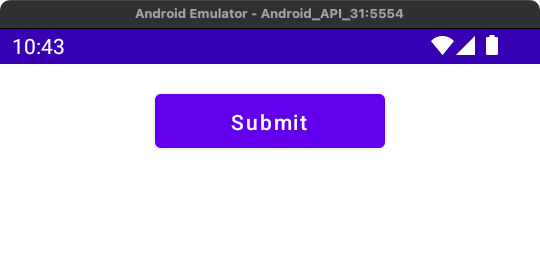
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set content padding for a Button using contentPadding parameter.