Android Compose – Invert colors of Image
In this tutorial, we will learn how to invert the colors of an image displayed in Image composable in Android Compose.
Image with original colors
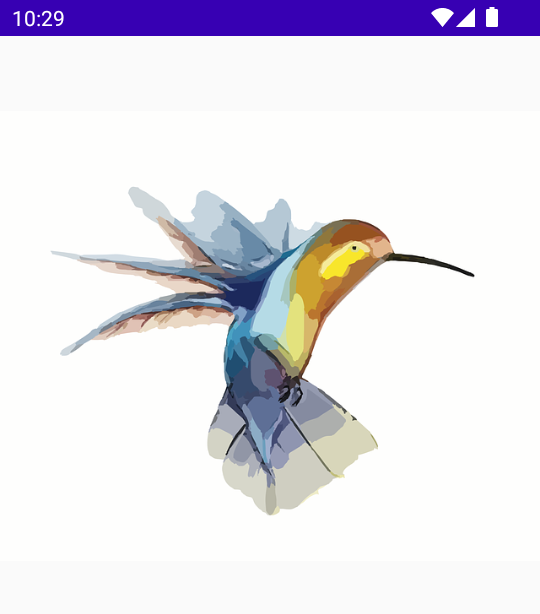
Image with inverted colors
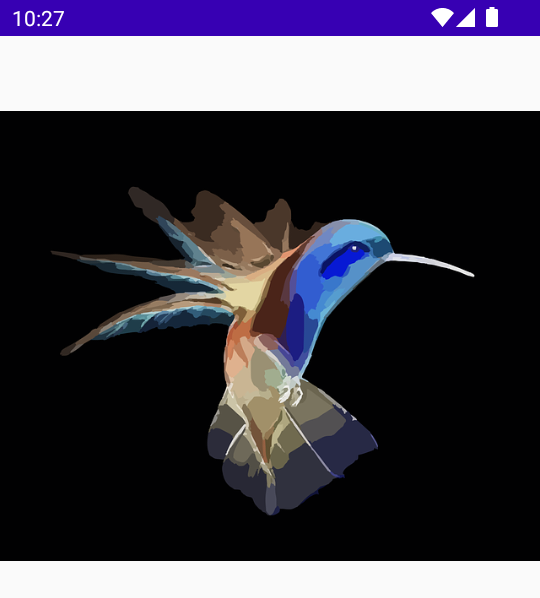
To invert the colors of an image in an Image composable in Android Jetpack Compose, use colorFilter parameter. Set the color matrix with values such that the contrast is inverted for the three color channels, thus inverting the colors of the image.
val colorMatrix = floatArrayOf(
-1f, 0f, 0f, 0f, 255f,
0f, -1f, 0f, 0f, 255f,
0f, 0f, -1f, 0f, 255f,
0f, 0f, 0f, 1f, 0f
)
Image(
painter = painterResource(id = R.drawable.hummingbird),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix))
)
where the colorMatrix represents the following values.
val colorMatrix = floatArrayOf(
contrast, 0f, 0f, 0f, brightness,
0f, contrast, 0f, 0f, brightness,
0f, 0f, contrast, 0f, brightness,
0f, 0f, 0f, 1f, 0f
)
So, we have assigned -1f
for contrast, and 255f
for brightness to invert the colors of the image.
Example
In this example, we have Compose Activity with an Image composable. We invert the colors of this image by using the above specified filter.
Screenshot – Image with original colors
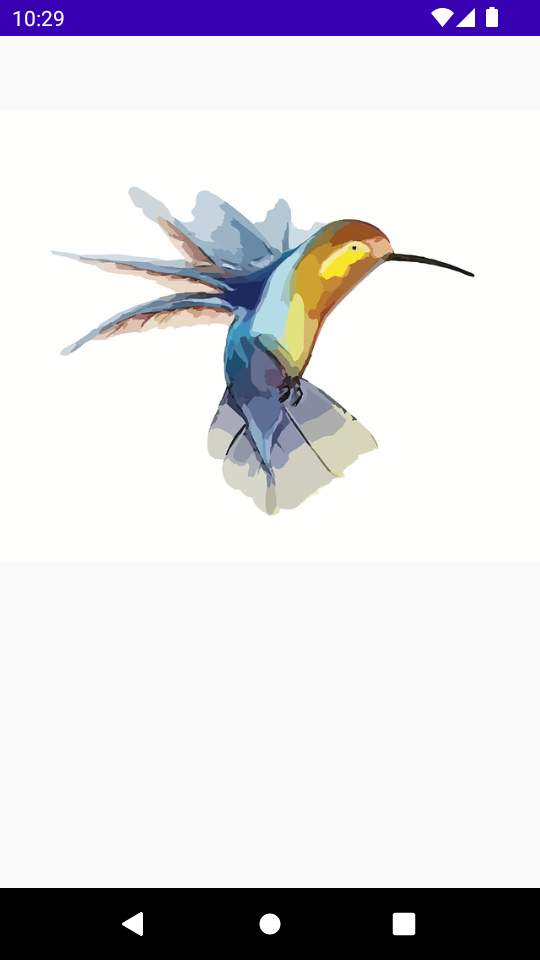
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.ColorFilter
import androidx.compose.ui.graphics.ColorMatrix
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
val colorMatrix = floatArrayOf(
-1f, 0f, 0f, 0f, 255f,
0f, -1f, 0f, 0f, 255f,
0f, 0f, -1f, 0f, 255f,
0f, 0f, 0f, 1f, 0f
)
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier.fillMaxWidth(), content = {
Spacer(modifier = Modifier.height(50.dp))
Image(
painter = painterResource(id = R.drawable.hummingbird),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix)),
)
}
)
}
}
}
}
Screenshot – Image with inverted colors
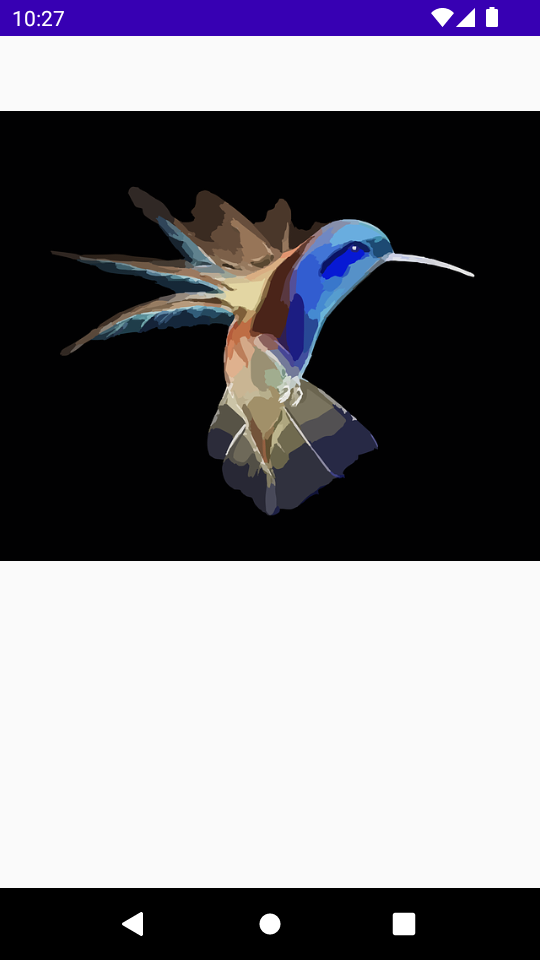
Project Download
The complete Android Studio Project in the above example is available for download at the following link. Download the ZIP file, uncompress it, and open with Android Studio.
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to invert colors of an Image composable by applying a filter.