Android Compose – Set Transparency for Image
To set alpha or transparency for Image in Android Compose, assign required alpha value for alpha
parameter of Image. alpha
parameter of Image accepts a float value between 0.0 and 1.0 corresponding to full transparency to no transparency, respectively.
The following code snippet shows how to set alpha or transparency for Image with 0.2
.
Image(
painter = painterResource(id = R.drawable.flower),
contentDescription = null,
alpha = 0.2F,
)
Example
In this example, let us display two Image views. The first Image will have a alpha of 0.2 (transparency of 80%) and the second Image will have a default alpha, which is 1.0 (transparency of 0%).
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier.fillMaxWidth(), content = {
Spacer(modifier = Modifier.height(10.dp))
Image(
painter = painterResource(id = R.drawable.flower),
contentDescription = null,
alpha = 0.2F,
)
Spacer(modifier = Modifier.height(10.dp))
Image(
painter = painterResource(id = R.drawable.flower),
contentDescription = null,
)
}
)
}
}
}
}
Screenshot
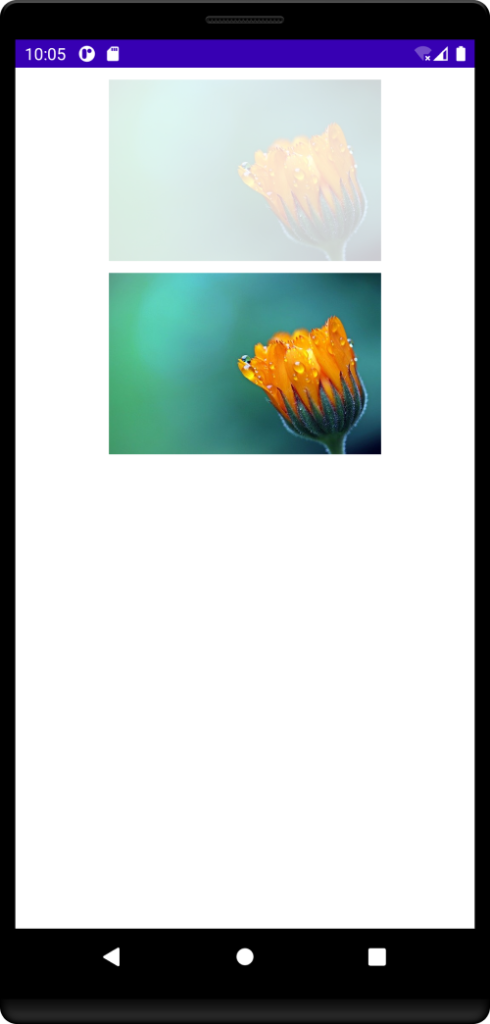
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set the alpha or transparency for Image, using alpha
parameter.