Android Jetpack Compose – TextField
TextField composable function is used to allow user to enter or modify text, in Android application.
The most basic example for a TextField composable, with value stored in a variable on any change in the value, a label, and an initial value displayed, is shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.material.Text import androidx.compose.material.TextField import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.runtime.remember import androidx.compose.runtime.getValue import androidx.compose.runtime.setValue import androidx.compose.runtime.mutableStateOf import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth() ) { var text by remember { mutableStateOf("Hello") } TextField( value = text, onValueChange = { text = it }, label = { Text("Label") } ) } } } } }
Screenshot
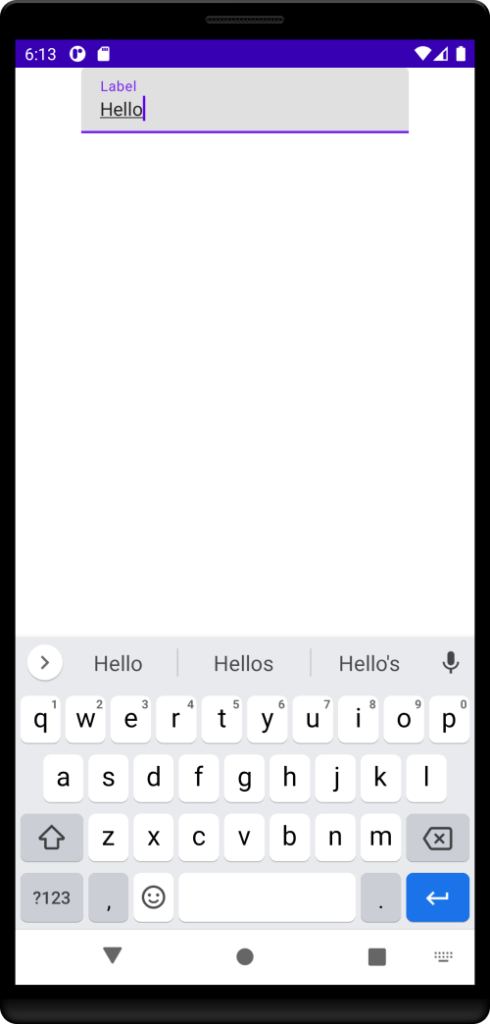
We also have an outlined version of this TextField, which is OutlinedTextField.
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.material.OutlinedTextField import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.runtime.remember import androidx.compose.runtime.getValue import androidx.compose.runtime.setValue import androidx.compose.runtime.mutableStateOf import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth() ) { var text by remember { mutableStateOf("Hello") } OutlinedTextField( value = text, onValueChange = { text = it }, label = { Text("Label") } ) } } } } }
Screenshot
ADVERTISEMENT
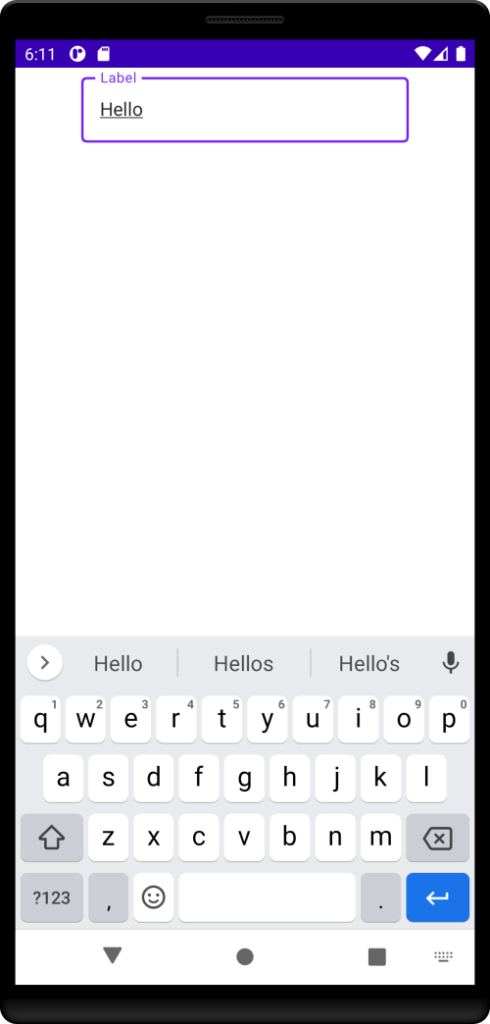
The following is a list of tutorials, that cover styling and how-to concepts with Text composable.