Android Compose – Set Height for Text
To set height for Text composable, in Android Jetpack Compose, assign modifier parameter of Text with Modifier companion object, where height() method is called on the Modifier. Pass required height value in the height() function call.
The following is a sample code snippet to set the heigth of Text composable.
import androidx.compose.foundation.layout.height import androidx.compose.ui.Modifier import androidx.compose.ui.unit.dp Text( "Hello World", modifier = Modifier.height(90.dp) )
Make sure to import height, Modifier and dp.
Example
Create an Android Application with Jetpack Compose as template, and modify MainActivity.kt as shown in the following.
In the following activity, the height of Text composable is set to 90dp.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.foundation.layout.height import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.unit.dp import androidx.compose.ui.unit.sp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth() ) { Text( "Hello World! This is a sample text. This is a sample text. This is a sample text.", fontSize = 30.sp, modifier = Modifier.height(90.dp) ) } } } } }
Screenshot in Emulator
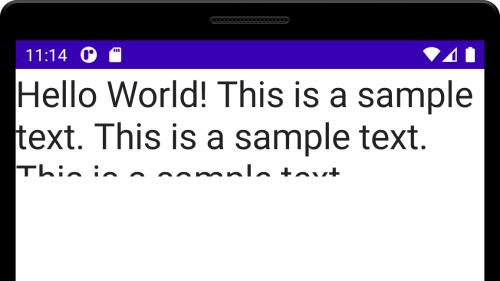
Let us define two Text composables, with different height values, say 50dp and 80dp.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.Spacer import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.foundation.layout.height import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.unit.dp import androidx.compose.ui.unit.sp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth() ) { Text( "Hello World! This is a sample text. This is a sample text. This is a sample text.", fontSize = 25.sp, modifier = Modifier.height(50.dp) ) Spacer(modifier = Modifier.height(20.dp)) Text( "Hello World! This is a sample text. This is a sample text. This is a sample text.", fontSize = 25.sp, modifier = Modifier.height(80.dp) ) } } } } }
Screenshot in Emulator
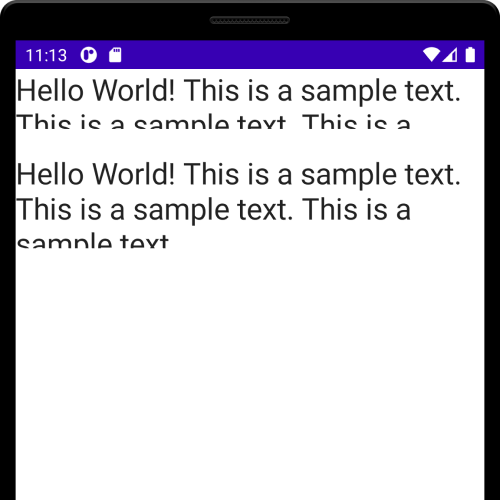
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set height for Text composable in Android Project with Jetpack Compose.