Android Compose – Button Shape
In this tutorial, we will learn how to set the shape of a Button in Android Compose.
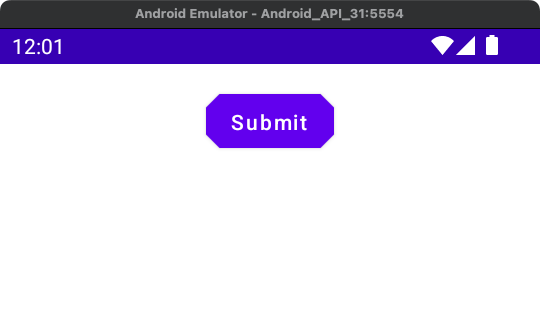
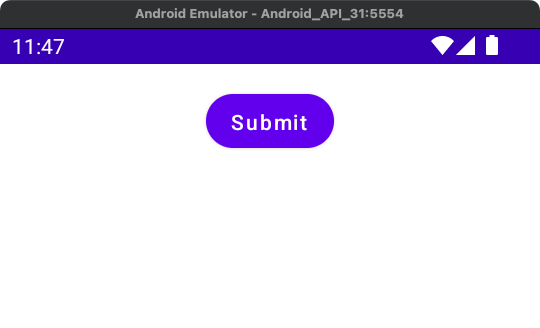
To set the shape of a Button in Android Jetpack Compose, set shape parameter of the Button with the required Shape object.
Button( shape = CircleShape, onClick = {}, ) { Text("Submit") }
Example
In this example, we have UI with a Button. The button has the text Submit
. And we set the shape of this Button to CircularShape.
Create a Project in Android Studio with empty compose activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import androidx.compose.foundation.layout.* import androidx.compose.foundation.shape.CircleShape import androidx.compose.material.Button import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier .padding(20.dp) .fillMaxWidth()) { Button( shape = CircleShape, onClick = {}, ) { Text("Submit") } } } } } }
Screenshot
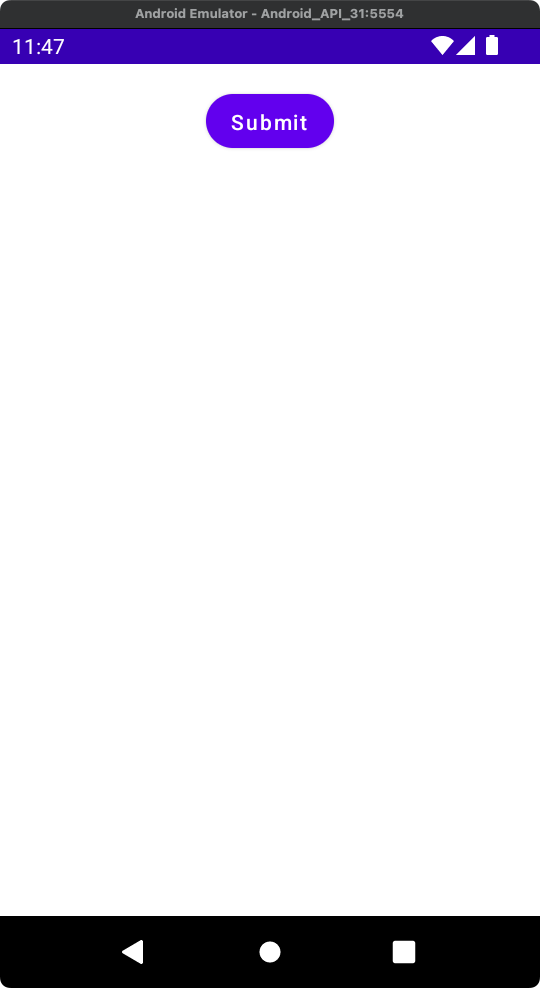
Button – Rectangle Shape
Now, let us change the shape of the button to rectangle by assigning shape parameter with RectangleShape.
Code for Button
Button( shape = RectangleShape, onClick = {}, ) { Text("Submit") }
Screenshot
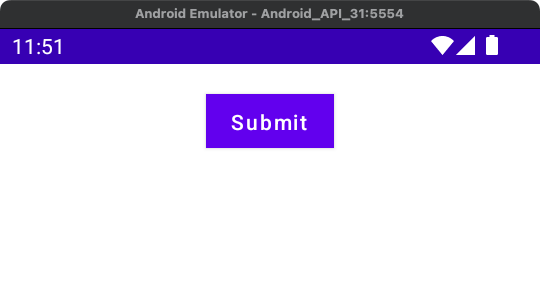
Button – Cut Corner Shape
To change the shape of the button to “Cut Corner”, assign shape parameter with CutCornerShape object with a specific percent of cut from the corners.
Code for Button
Button( shape = CutCornerShape(percent = 25), onClick = {}, ) { Text("Submit") }
Screenshot
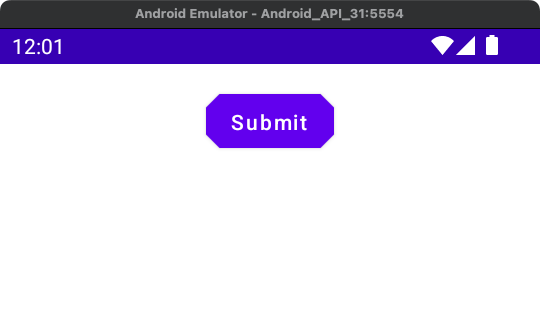
Button – Rounded Corner Shape
Now, let us change the shape of the button to rounded corners by assigning shape parameter with RoundedCornerShape object with corners rounded to specified length.
Code for Button
Button( shape = RoundedCornerShape(10.dp), onClick = {}, ) { Text("Submit") }
Screenshot
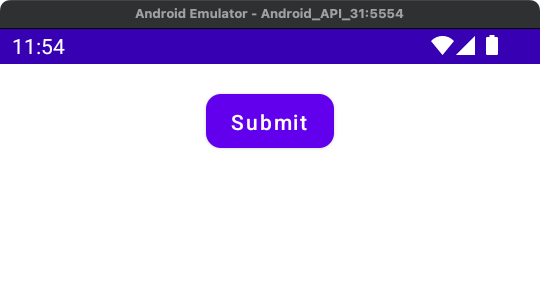
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set shape of a Button using its shape parameter.