Android Compose – Set Font Weight for Text
To set font weight of Text composable, in Android Jetpack Compose, pass required FontWeight
value for the optional fontWeight
parameter of Text composable.
The following is a sample code snippet to set the width of Text composable.
import androidx.compose.ui.text.font.FontWeight Text( "Hello World", fontWeight = FontWeight.Bold )
Make sure to include FontWeight in the imports.
Example
Create an Android Application with Jetpack Compose as template, and modify MainActivity.kt as shown in the following.
In the following activity, the font weight of Text composable is set to extra bold.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.text.font.FontWeight import androidx.compose.ui.unit.sp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth() ) { Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.ExtraBold ) } } } } }
Screenshot in Emulator
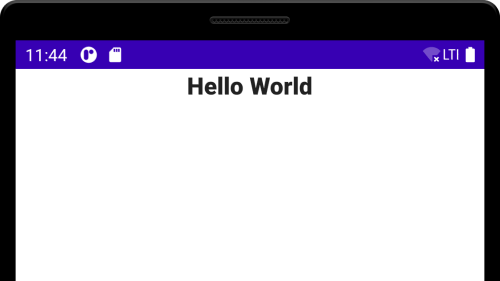
Let us define different Text composables, with different font weight values like
- FontWeight.W100
- FontWeight.W200
- FontWeight.W300
- FontWeight.W400
- FontWeight.W500
- FontWeight.W600
- FontWeight.W700
- FontWeight.W800
- FontWeight.W900
Or you can use the following values, where are the respective aliases for the above list of font weight values.
- FontWeight.Thin
- FontWeight.ExtraLight
- FontWeight.Light
- FontWeight.Normal
- FontWeight.Medium
- FontWeight.SemiBold
- FontWeight.Bold
- FontWeight.ExtraBold
- FontWeight.Black
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.text.font.FontWeight import androidx.compose.ui.unit.sp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth() ) { Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.Thin ) Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.ExtraLight ) Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.Light ) Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.Normal ) Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.Medium ) Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.SemiBold ) Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.W900 ) Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.ExtraBold ) Text( "Hello World", fontSize = 20.sp, fontWeight = FontWeight.Black ) } } } } }
Screenshot in Emulator
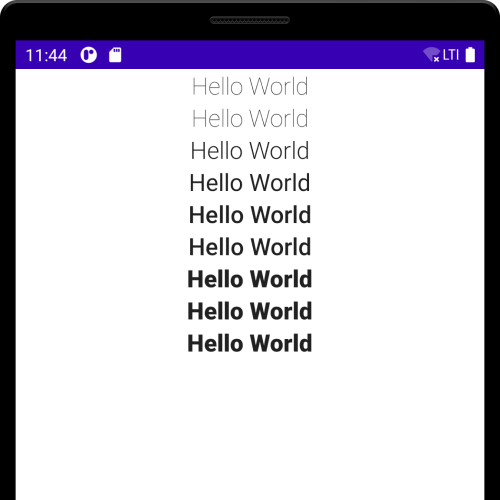
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set font weight for Text composable in Android Project with Jetpack Compose.