Spacer – Fill Maximum Width
To set Spacer component to fill maximum width allowed by its parent, call fillMaxWidth() on the Modifier object of Spacer.
The following code snippet gives a quick look on how to call fillMaxWidth() on the Modifier object.
Spacer(
modifier = Modifier
.fillMaxWidth()
)
Example
In this example, we will display two Rows, and between them we place a Spacer with a height of 20dp. The width of this Spacer will be set to fill the maximum width allowed by its parent, which is the Column.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material.Scaffold
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Scaffold(
topBar = {
TopAppBar(title = {Text("Spacer Example - TutorialKart")})
}
) {
Column(Modifier.padding(5.dp)) {
Row(
modifier = Modifier
.height(50.dp)
.fillMaxWidth()
.background(Color.Gray)
) {}
Spacer(
modifier = Modifier
.fillMaxWidth()
.height(20.dp)
.background(Color.Blue)
)
Row(
modifier = Modifier
.height(50.dp)
.fillMaxWidth()
.background(Color.Gray)
) {}
}
}
}
}
}
}
Screenshot
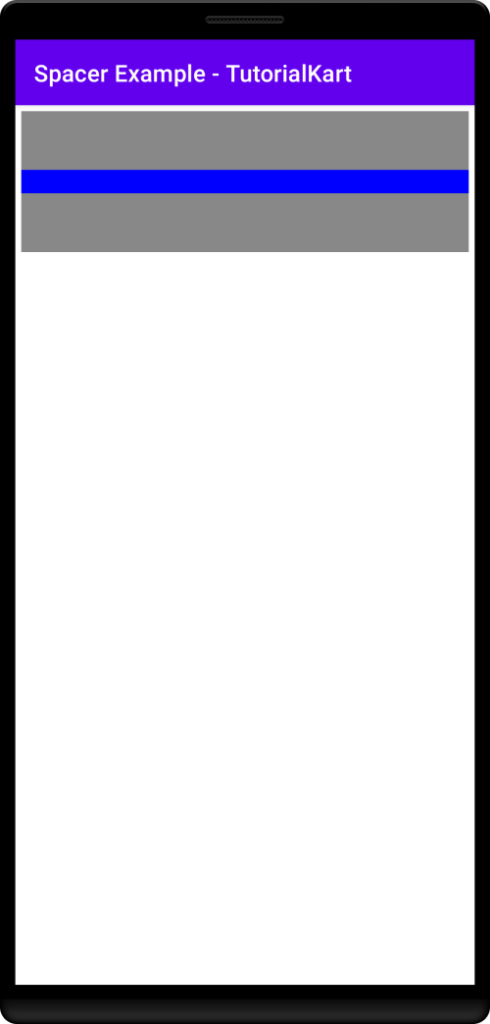
Now, let us check how Spacer displays when width is set to specific value, say 100dp.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material.Scaffold
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Scaffold(
topBar = {
TopAppBar(title = {Text("Spacer Example - TutorialKart")})
}
) {
Column(Modifier.padding(5.dp)) {
Row(
modifier = Modifier
.height(50.dp)
.fillMaxWidth()
.background(Color.Gray)
) {}
Spacer(
modifier = Modifier
.width(100.dp)
.height(20.dp)
.background(Color.Blue)
)
Row(
modifier = Modifier
.height(50.dp)
.fillMaxWidth()
.background(Color.Gray)
) {}
}
}
}
}
}
}
Screenshot
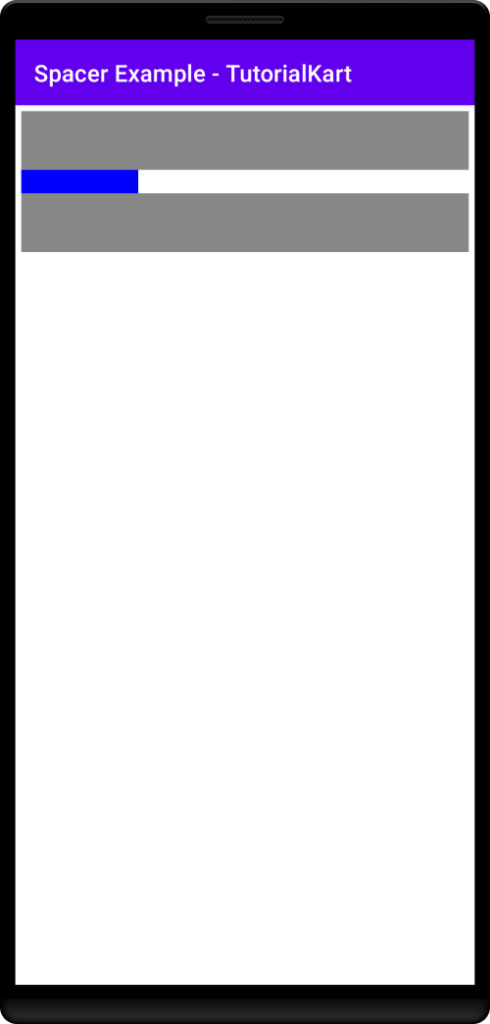
We can compare the result of fillMaxWidth() modifier function against setting specific width to the spacer.
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set Spacer to fill maximum width allowed by its parent, in Android Jetpack Compose.