In this tutorial, you will learn how to check if a given directory is empty or not in Swift language using contentsOfDirectory() method.
Check if given Directory is Empty in Swift
To check if a given directory is empty or not, in Swift, we have to check if the contents of the directory is empty or not.
Follow these steps.
- Given a path to directory.
- Get the default shared File manager using FileManager.defaut.
- Get the contents of the directory by calling contentsOfDirectory() method of file manager, and passing the path to directory.
- Read the isEmpty property of the contents returned.
- If isEmpty property returns true, then the given directory is empty. Or else, the directory is not empty.
- We can use the isEmpty property as condition in Swift if-else statement.
The syntax to get the contents of the directory at path is
fileManager.contentsOfDirectory(atPath: path)
Examples
1. Check if directory is empty
In this example, we take a path "/Users/tutorialkart/dummy/"
, and check if this directory is empty or not.
The given directory is empty.

main.swift
import Foundation let path = "/Users/tutorialkart/dummy/" let fileManager = FileManager.default do { let contents = try fileManager.contentsOfDirectory(atPath: path) if contents.isEmpty { print("DIRECTORY IS EMPTY.") } else { print("DIRECTORY IS NOT EMPTY.") } } catch { print("Error reading directory contents: \(error)") }
Output
Since the given directory is empty, isEmpty property returns true, and the if-block is run.
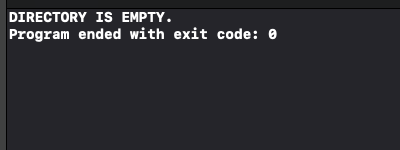
2. Directory is not empty
In this example, we take a path "/Users/tutorialkart/"
, and check if this directory is empty or not.
main.swift
import Foundation let path = "/Users/tutorialkart/" let fileManager = FileManager.default do { let contents = try fileManager.contentsOfDirectory(atPath: path) if contents.isEmpty { print("DIRECTORY IS EMPTY.") } else { print("DIRECTORY IS NOT EMPTY.") } } catch { print("Error reading directory contents: \(error)") }
Output
The directory at given path is not empty, and therefore, isEmpty property returns false, and the else-block is run.
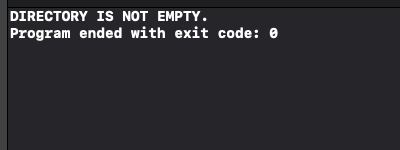
Conclusion
In this Swift Tutorial, we have seen how to check if a directory is empty or not, using contentsOfDirectory() method of file manager, with examples.