In this tutorial, you will learn how to check if a file at given file path is empty or not in Swift language using file size attribute.
Check if a File is Empty in Swift
To check if a file is empty, at a given file path, in Swift, follow these steps.
- Given a path to the file.
- Get the default shared File manager using FileManager.defaut.
- Get attributes of the file at given path using attributesOfItem() method of the file manager. We have to pass the file path to the attributesOfItem() method as argument.
- From the attributes, get the size attribute.
- If the size is zero, then the file is empty.
- Or if the size is not zero, then the file is not empty.
- You can use a Swift if-else statement with the file size equals 0 as if-condition.
Examples
1. Empty file scenario – Check if file at given path is empty
In this example, we take a file path "/Users/tutorialkart/nothing.txt"
, and check if the file is empty at given path, using size file attribute.

From the above screenshot, the file size is 0 bytes.
We have taken the file path such that the file is empty. Therefore, the size attribute returns zero, and the if-block should execute.
main.swift
import Foundation
let filePath = "/Users/tutorialkart/nothing.txt"
let fileManager = FileManager.default
let attribtues = try fileManager.attributesOfItem(atPath: filePath)
let fileSize = attribtues[.size] as? Int
print("File size : \(fileSize!)")
if fileSize == 0 {
print("FILE IS EMPTY.")
} else {
print("FILE IS NOT EMPTY.")
}
Output
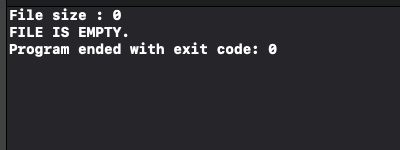
2. Non-empty file scenario
In this example, we take a file path "/Users/tutorialkart/data.txt"
, and check if the file is empty at given path, using size file attribute.

From the above screenshot, the file size is 51 bytes.
We have taken the file path such that the file is not empty. Therefore, the size attribute returns a non-zero value, and the else-block should execute.
main.swift
import Foundation
let filePath = "/Users/tutorialkart/data.txt"
let fileManager = FileManager.default
let attribtues = try fileManager.attributesOfItem(atPath: filePath)
let fileSize = attribtues[.size] as? Int
print("File size : \(fileSize!)")
if fileSize == 0 {
print("FILE IS EMPTY.")
} else {
print("FILE IS NOT EMPTY.")
}
Output
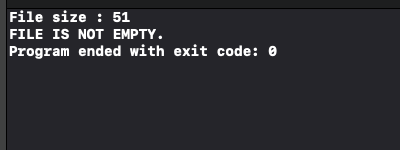
Conclusion
In this Swift Tutorial, we have seen how to check if a file is empty or not, using file size attribute, with examples.