Read file line by line in Swift
To read a file line by line in Swift,
- Given path to the file.
- Use freopen() method and open the file at given path in read mode, and associate the file stream with the standard input stdin.
- Now, that the standard input stream is set with the file contents, you can read the lines from the file using readLine() method, just like you read a line from standard input.
- You can use while loop to read all the lines one by one, until there are none.
Examples
1. Read file line by line
In this example, we take a string value in originalString, and replace the search character "o"
with the replacement character "X"
.
main.swift
</>
Copy
import Foundation
let path = "/Users/tutorialkart/data.txt"
guard let file = freopen(path, "r", stdin) else {
print("File not present.")
throw NSError()
}
var i = 0
while let line = readLine() {
i += 1
print("Line \(i) - \(line)")
}
In the program,
- path variable is set with the path to the file.
- freopen() method is used to read the file at the path and associate the file stream to the stdin. If there is an error with opening the file, we shall print a message, and throw NSError. You may modify this else part, based on your file handling requirements.
- A while loop is used to read the lines from standard input using readLine() method. Inside the while loop, we used a counter variable i, to print the line numbers.
Replace the path to the file, with the path to the file from your system.
Output
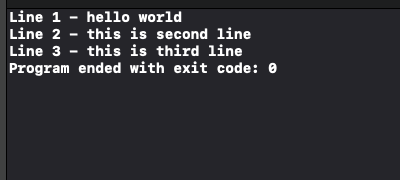
Conclusion
In this Swift Tutorial, we have seen how to read a file at given path, line by line, with examples.