Flutter – Banner Location
To set specific location for a Banner widget in Flutter, set location property with any of the following values.
- BannerLocation.topStart
- BannerLocation.topEnd
- BannerLocation.bottomStart
- BannerLocation.bottomEnd
Code Snippet
The following is a quick code snippet to set a specific location for message in a Banner widget
</>
Copy
Banner(
location: BannerLocation.topStart,
)
Example
In the following example, we create a Flutter Application with four Banner widgets. Each of the Banner widget is set with one of the possible location values.
main.dart
</>
Copy
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Tutorial';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
@override
Widget build(BuildContext context) {
return Center(
child: Column(
children: <Widget>[
const SizedBox(height: 10,),
Banner(
message: 'New Arrival',
location: BannerLocation.topStart,
child: Container(
height: 150,
width: 150,
color: Colors.yellow,
alignment: Alignment.center,
child: const Text('Item 1'),
),
),
const SizedBox(height: 10,),
Banner(
message: 'New Arrival',
location: BannerLocation.topEnd,
child: Container(
height: 150,
width: 150,
color: Colors.yellow,
alignment: Alignment.center,
child: const Text('Item 2'),
),
),
const SizedBox(height: 10,),
Banner(
message: 'New Arrival',
location: BannerLocation.bottomStart,
child: Container(
height: 150,
width: 150,
color: Colors.yellow,
alignment: Alignment.center,
child: const Text('Item 3'),
),
),
const SizedBox(height: 10,),
Banner(
message: 'New Arrival',
location: BannerLocation.bottomEnd,
child: Container(
height: 150,
width: 150,
color: Colors.yellow,
alignment: Alignment.center,
child: const Text('Item 4'),
),
),
],
),
);
}
}
Screenshot – iPhone Simulator
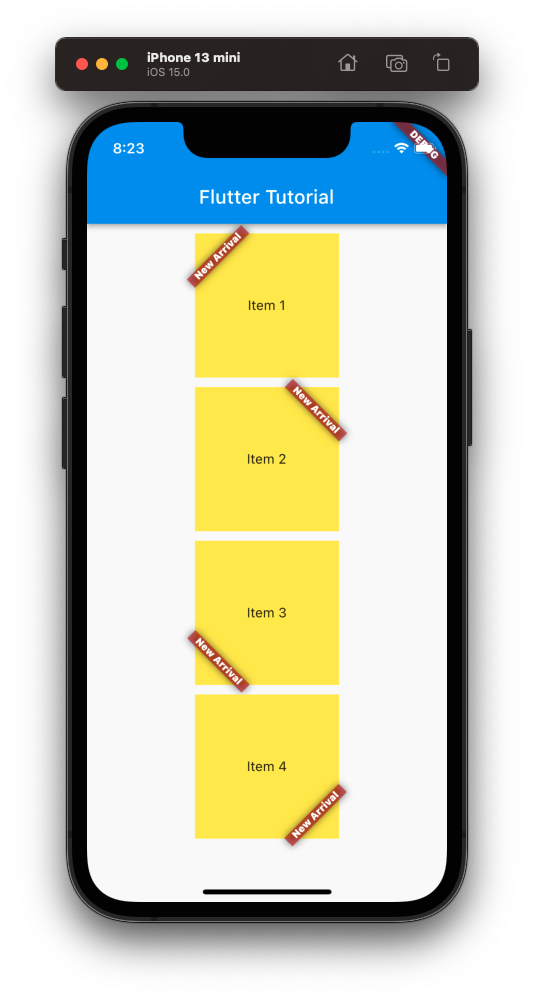
Screenshot – Android Emulator
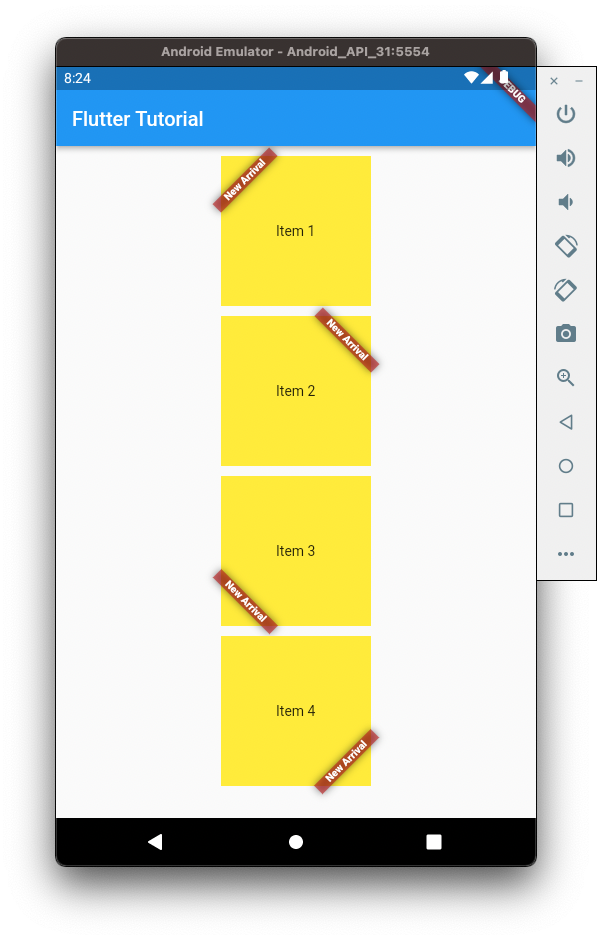
Conclusion
In this Flutter Tutorial, we learned how to set specific location for the banner message in Banner widget, with examples.