Flutter SnackBar
SnackBar is a widget to show a lightweight message at the bottom of screen. It can also contain an optional action.
SnackBar is usually used with Scaffold and the usage is shown in the example below.
Example
In this tutorial, we will go through an example, where we have two buttons. When a button is clicked, it calls a function that shows SnackBar at the bottom of the screen.
main.dart
</>
Copy
import 'package:flutter/material.dart';
void main() {
runApp(MaterialApp(
home: MyApp(),
));
}
class MyApp extends StatefulWidget {
@override
_State createState() => _State();
}
class _State extends State<MyApp> {
final GlobalKey<ScaffoldState> _scaffoldKey = new GlobalKey<ScaffoldState>();
void _showScaffold(String message) {
_scaffoldKey.currentState.showSnackBar(SnackBar(
content: Text(message),
));
}
@override
Widget build(BuildContext context) {
return Scaffold(
key: _scaffoldKey,
appBar: AppBar(
title: Text('Flutter - tutorialkart.com'),
),
body: Center(
child: Column(children: <Widget>[
RaisedButton(
textColor: Colors.white,
color: Colors.green,
child: Text('Show SnackBar'),
onPressed: () {
_showScaffold("This is a SnackBar.");
},
),
RaisedButton(
textColor: Colors.white,
color: Colors.deepPurpleAccent,
child: Text('Show SnackBar 1'),
onPressed: () {
_showScaffold("This is a SnackBar called from another place.");
},
),
])));
}
}
When you run this Flutter application, you will get UI as shown below with two buttons.
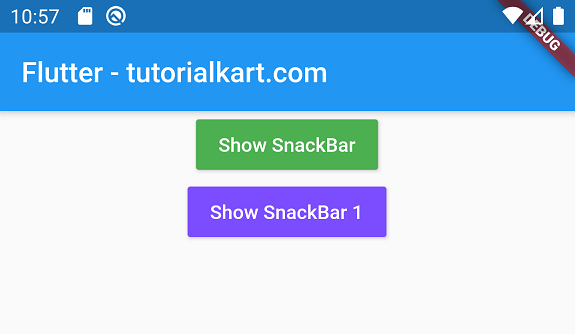
When you click on a button, SnackBar is shown at the bottom of screen as shown below.
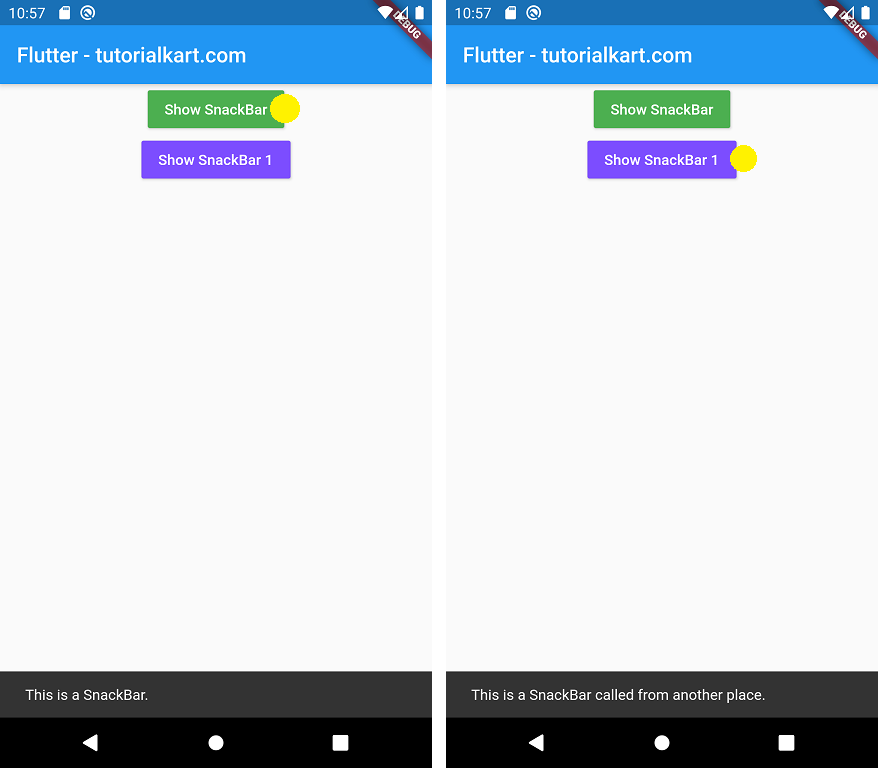
Conclusion
In this Flutter Tutorial, we learned about SnackBar and how to use it using Flutter Example Application.