Flutter – RaisedButton Color property not working
In this tutorial, we will solve the problem of Flutter RaisedButton where changing its color using color
property does not affect in UI.
Solution
The solution is adding onPressed() property to RaisedButton.
Because, if onPressed() property is not provided, even with the empty function, RaisedButton is considered as disabled. Hence grey colored button appears, even if we provide a color to Raised Button.
Example with onPressed() not given
In the following example, we will define a RaisedButton in our UI, with color property given, but without onPressed().
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Center(child: Text('Flutter - tutorialkart.com')),
),
body: Container(
child: ListView(children: <Widget>[
Container(
child: RaisedButton(
textColor: Colors.white,
color: Colors.lightGreen,
child: Text(
'Click Me',
style: TextStyle(fontSize: 20),
))),
])),
));
}
}
When you run this application, the RaisedButton appears in grey, despite of the color property provided.
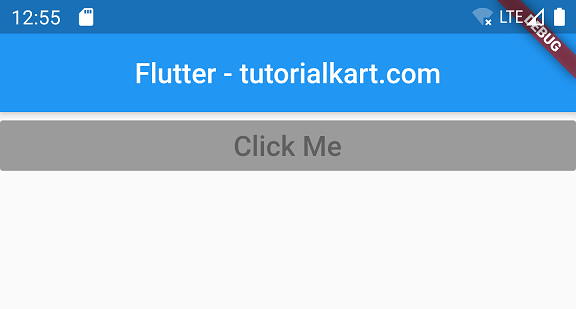
Example with onPressed property given
Now, add onPressed property to RaisedButton as shown below.
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Center(child: Text('Flutter - tutorialkart.com')),
),
body: Container(
child: ListView(children: <Widget>[
Container(
child: RaisedButton(
onPressed: () => {},
textColor: Colors.white,
color: Colors.lightGreen,
child: Text(
'Click Me',
style: TextStyle(fontSize: 20),
))),
])),
));
}
}
When we run this application, we get RaisedButton with the color provided, since the button is in enabled state because of onPressed property.
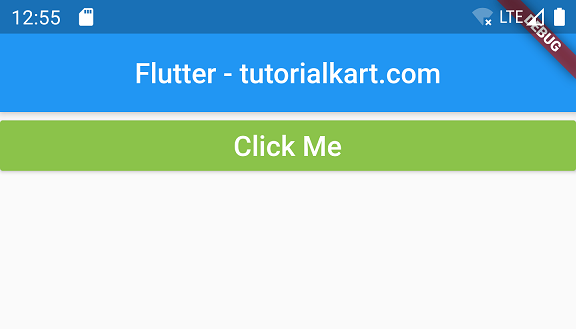
Conclusion
In this Flutter Tutorial, we learned how to enable RaisedButton widget and make color working for it.