Slider Widget – Active Color, Inactive Color, Thumb Color
We can change the active area of slider, inactive area of slider, and thumb color using activeColor
, inactiveColor
, and thumbColor
properties of Slider class respectively.
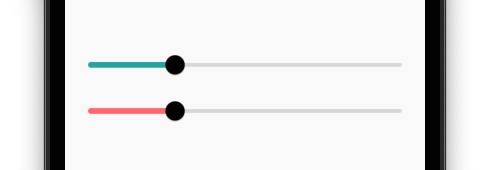
Syntax
The syntax of Slider widget with different color properties is
</>
Copy
Slider(
activeColor: Color.black,
inactiveColor: Color.black12,
thumbColor: Color.black38,
),
Example
In the following example, we create a Flutter Application, with active slider color, inactive slider color, and thumb color set with different Color values.
main.dart
</>
Copy
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Slider Tutorial';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
double sliderValue = 0.2;
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Slider(
value: sliderValue,
onChanged: (double value) {
setState(() {
sliderValue = value;
});
},
activeColor: const Color.fromARGB(255, 69, 173, 168),
inactiveColor: Colors.black12,
thumbColor: Colors.black,
),
]
);
}
}
Screenshot
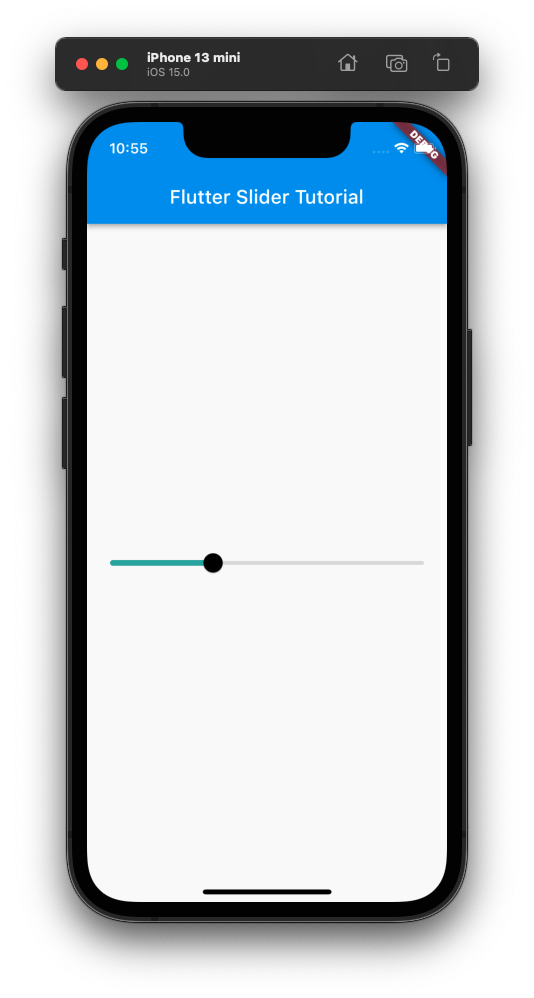
Conclusion
In this Flutter Tutorial, we learned how to change the colors of active area, inactive area, and thumb color of Slider widget, with examples.