Flutter Switch
Flutter Switch is used to toggle a setting between on/off which is true/false respectively.
When the switch is on, the value returned by the Switch onChanged property is true, while the switch is off, onChanged property returns false.
Example
In the following example Flutter application, we defined a Switch widget. Whenever the Switch is toggled, onChanged is called with new state of Switch as value.
We have defined a boolean variable isSwitched to store the state of Switch.
Create a basic Flutter application and replace main.dart with the following code.
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(MaterialApp(
home: MyApp(),
));
}
class MyApp extends StatefulWidget {
@override
_State createState() => _State();
}
class _State extends State<MyApp> {
bool isSwitched = false;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter - tutorialkart.com'),
),
body: Center(
child: Switch(
value: isSwitched,
onChanged: (value) {
setState(() {
isSwitched = value;
print(isSwitched);
});
},
activeTrackColor: Colors.lightGreenAccent,
activeColor: Colors.green,
),
)
);
}
}
Run this application and you should get the UI as shown in the following screenshot.
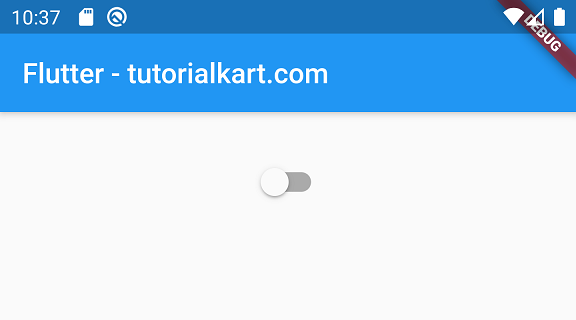
When you press on the Switch, the Switch toggles the state, from off to on and is displayed as shown below.
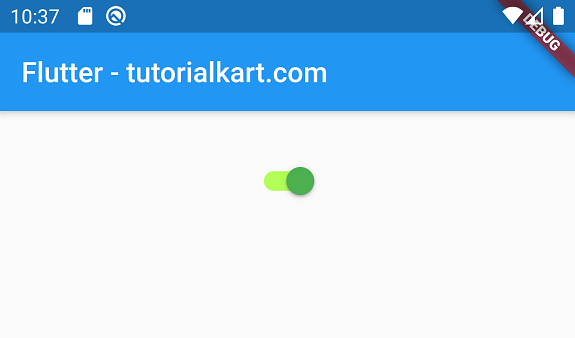
You can define the colors for a Switch as we have defined in this example.
Conclusion
In this Flutter Tutorial, we learned how to use Flutter Switch and access its value when the state is toggled.