Flutter – Container Border Radius
To set border radius for Container widget, set its decoration property with BoxDecoration where the borderRadius property is set with required value.
Code Snippet
A quick code snippet to set the border radius for a Container widget is
</>
Copy
Container(
decoration: const BoxDecoration(
borderRadius: BorderRadius.all(Radius.circular(15)),
),
)
Example
In the following example, we create a Flutter Application with two Container widgets. The first Container widget is set with a border radius of 15, and the second Container widget is set with a border radius of 25.
main.dart
</>
Copy
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Tutorial';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
@override
Widget build(BuildContext context) {
return Center(
child: Column(
children: <Widget>[
const SizedBox(height: 10,),
Container(
height: 150,
width: 150,
decoration: const BoxDecoration(
borderRadius: BorderRadius.all(Radius.circular(15)),
color: Colors.lime,
),
alignment: Alignment.center,
child: const Text('Radius 15'),
),
const SizedBox(height: 10,),
Container(
height: 150,
width: 150,
decoration: const BoxDecoration(
borderRadius: BorderRadius.all(Radius.circular(25)),
color: Colors.lime,
),
alignment: Alignment.center,
child: const Text('Radius 25'),
),
],
),
);
}
}
Screenshot – iPhone Simulator
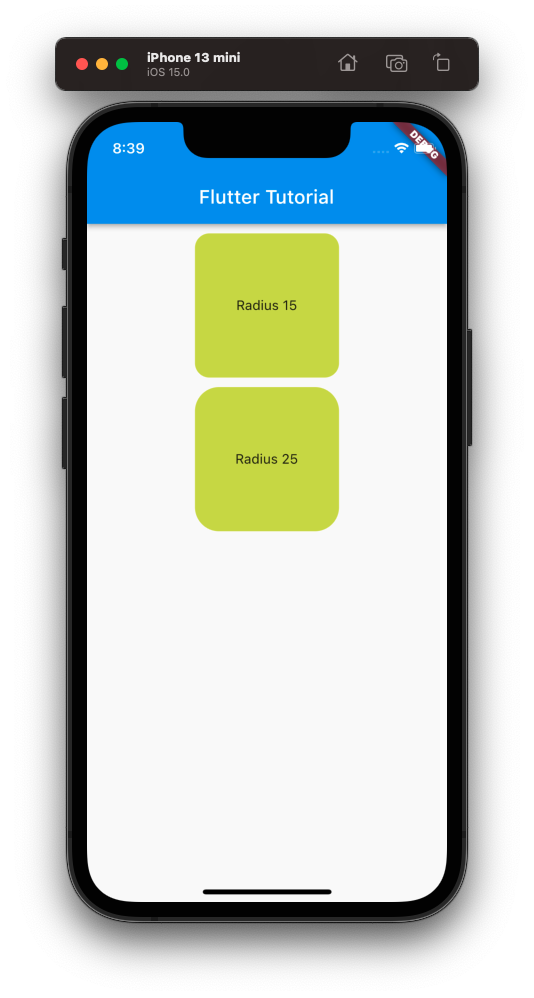
Screenshot – Android Emulator
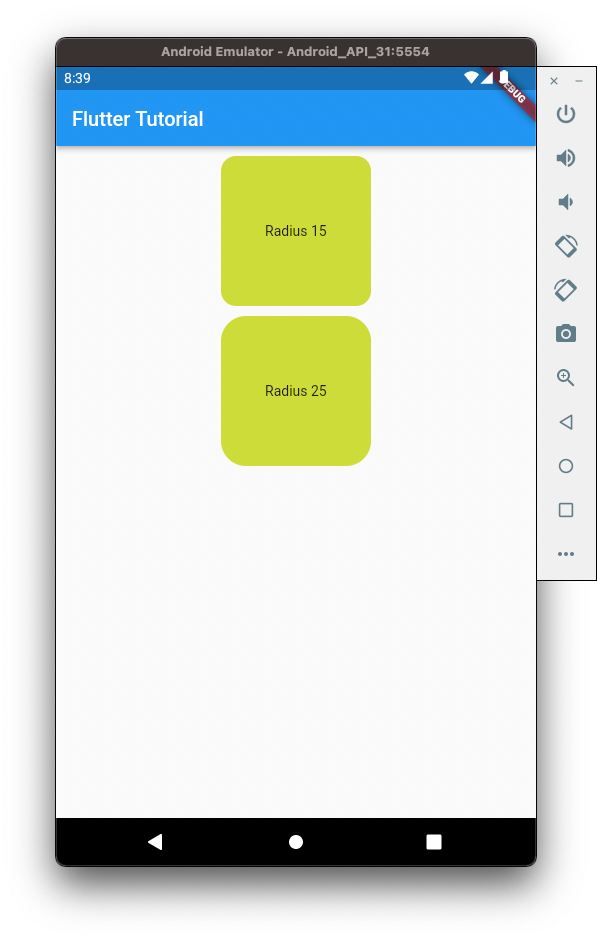
Conclusion
In this Flutter Tutorial, we learned how to set specific location for the banner message in Banner widget, with examples.