Flutter Image – Rounded Corners
To display an image with rounded corners or circular shaped corners, place the Image widget as child of ClipRRect widget with circular border radius specified for the ClipRRect widget.
Let us learn briefly what this ClipRRect is. ClipRRect is a Flutter widget that clips its child with a rounded rectangle. You can specify a specific border radius for this widget, specifically circular border radius, using borderRadius property.
Now, to display an image with rounded corners, follow the below sequence of steps in your application code.
- Surround your Image widget with ClipRRect widget.
- Specify the required border radius. As we need rounded borders, we specify circular border radius using BorderRadius.circular().
- BorderRadius.circular() takes a double value as argument. This double value is the border radius for all the four corners of the rectangle.
Following is the code snippet to display an image with round corners.
ClipRRect( borderRadius: BorderRadius.circular(20), child: Image( image: NetworkImage( 'https://www.tutorialkart.com/img/hummingbird.png'), ), ),
The source of your image is your choice. NetworkImage is only for displaying an image. You can use an image from assets folder as well.
Example – Flutter Image with Rounded Corners
In this example, we have two images with rounded corners. Let us first run the application, and go through the code in detail.
main.dart
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatefulWidget { @override _MyAppState createState() => _MyAppState(); } class _MyAppState extends State<MyApp> { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter Image - tutorialkart.com'), ), body: Center( child: Container( color: Colors.cyan, width: double.infinity, child: Column(children: <Widget>[ Container( width: 200, margin: EdgeInsets.all(10), child: ClipRRect( borderRadius: BorderRadius.circular(20), child: Image( image: NetworkImage( 'https://www.tutorialkart.com/img/hummingbird.png'), ), ), ), Container( width: 200, margin: EdgeInsets.all(10), child: ClipRRect( borderRadius: BorderRadius.circular(50), child: Image( image: NetworkImage( 'https://www.tutorialkart.com/img/hummingbird.png'), ), ), ), ]), ), ), ), ); } }
First Image with Rounded Corners
For the first Image widget, we have a ClipRRect surrounding the image with a circular radius of 20
. So, ClipRRect clips its child, the image, with a rounded rectangle. The corners have a circular shape with radii of 20.
Second Image with Rounded Corners
For the second Image widget, we have a ClipRRect surrounding the image with a circular radius of 50
. So, ClipRRect clips its child, the image, with a rounded rectangle. The corners have a circular shape with radii of 50.
This circular radius is greater than that of used for first image. And is evident in the following screenshot.
Screenshot
Run the application in an Emulator or on a Original Device. You should get an application running with the screen displaying two images with round corners as shown below.
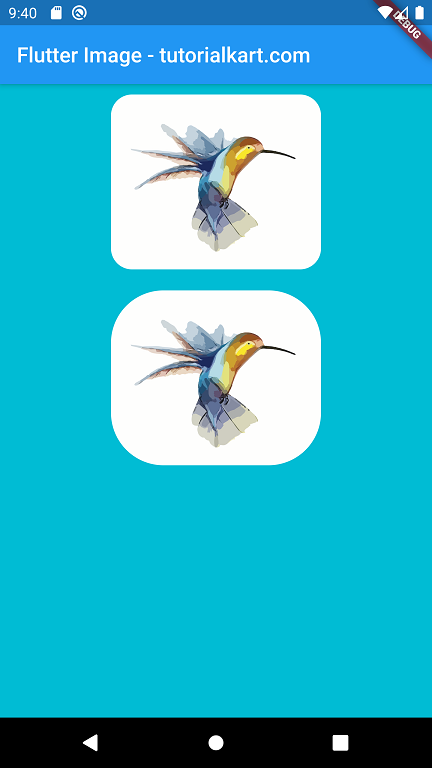
Conclusion
In this Flutter Tutorial, we learned how to clip the corners of an image using a ClipRRect widget to get the look of an image with rounded corners.