Flutter – Build Items of GridView Dynamically
To generate items in a GridView programmatically, use GridView.builder() constructor to create GridView. This constructor allows us to specify an item builder function, via itemBuilder
property, with the context and index of item as parameters. Also, we have to specify the number of items in the grid view via itemCount
property.
Code Snippet
The following is an example code snippet for GridView.builder(), with item builder function.
</>
Copy
GridView.builder(
gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 4
),
itemCount: 40,
itemBuilder: (BuildContext context, int index) {
return Card(
color: Colors.primaries[index % 10],
child: Center(child: Text('$index')),
);
},
);
Example
In the following example, we create a Flutter Application with a GridView. We dynamically generate 40 items in this GridView.
main.dart
</>
Copy
import 'dart:ui';
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Tutorial';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
@override
Widget build(BuildContext context) {
return GridView.builder(
gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 4
),
itemCount: 40,
itemBuilder: (BuildContext context, int index) {
return Card(
color: Colors.primaries[index % 10],
child: Center(child: Text('$index')),
);
},
);
}
}
Screenshot – iPhone Simulator
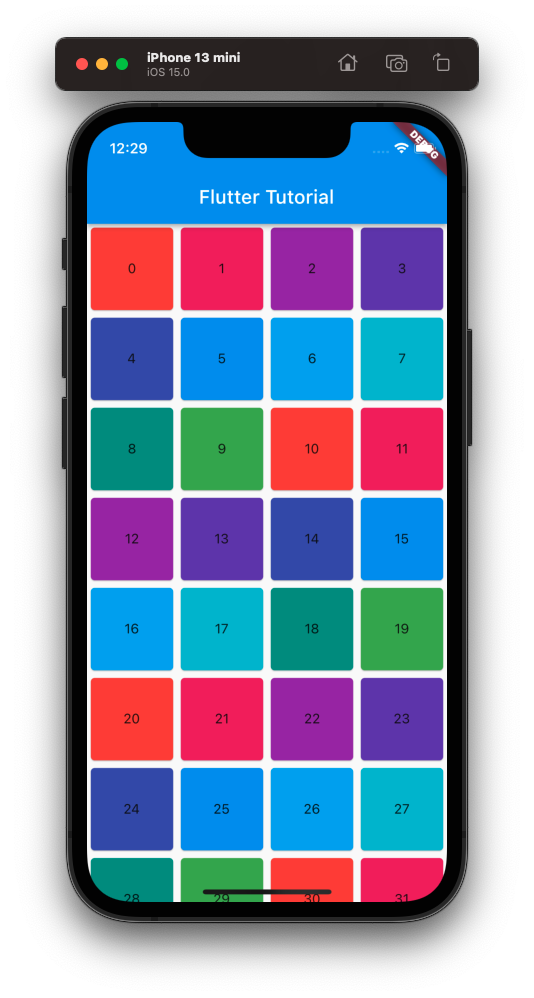
Screenshot – Android Emulator
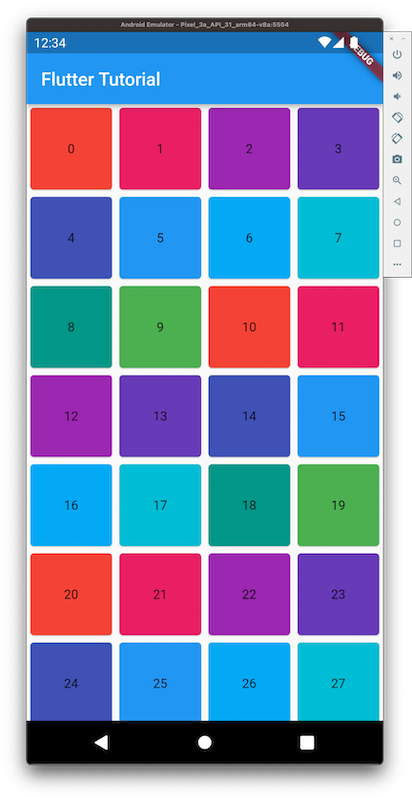
Conclusion
In this Flutter Tutorial, we learned how to dynamically build items in a GridView using itemBuilder, with examples.