Flutter ElevatedButton
Flutter ElevatedButton class is used to display at button with an elevation from the background/surrounding widget.
By default, when an Elevated button is pressed, the elevation of the button increases.
Syntax
The syntax to display an ElevatedButton widget with onPressed callback and text inside the button, is shown in the following.
ElevatedButton(
onPressed: () { },
child: const Text('Click Me'),
)
Example
In the following Flutter Application, we shall display an ElevatedButton with the text 'Click Me'
.
main.dart
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
/// main application widget
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Tutorial';
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: _title,
home: MyStatelessWidget(),
);
}
}
/// stateless widget that the main application instantiates
class MyStatelessWidget extends StatelessWidget {
const MyStatelessWidget({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('ElevatedButton Tutorial')),
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
onPressed: () { },
child: const Text('Click Me'),
),
],
),
),
);
}
}
Screenshot – Android Emulator
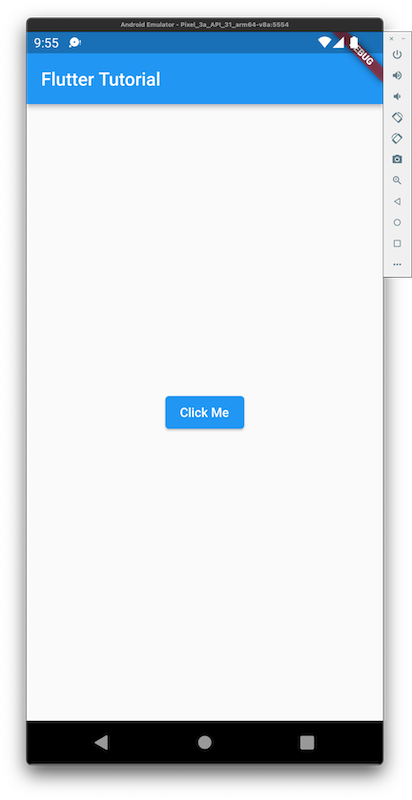
Screenshot – iPhone Simulator
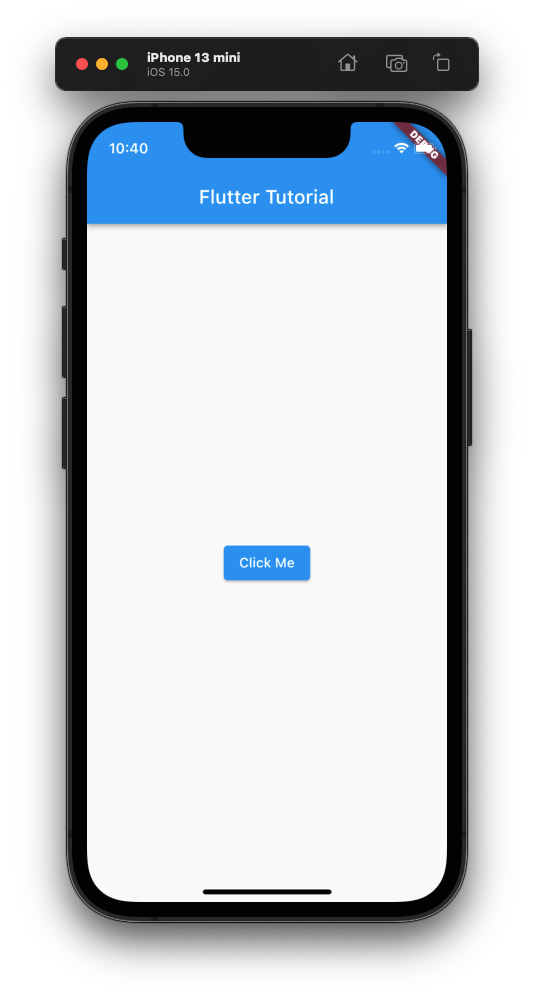
ElevatedButton onPressed
We can execute a function when ElevatedButton is pressed using onPressed
callback.
In the following Flutter Application, we shall display a SnackBar when ElevatedButton is pressed.
main.dart
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
/// main application widget
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Tutorial';
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: _title,
home: MyStatelessWidget(),
);
}
}
/// stateless widget that the main application instantiates
class MyStatelessWidget extends StatelessWidget {
const MyStatelessWidget({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('ElevatedButton Tutorial')),
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(content: Text('You pressed the button.')));
},
child: const Text('Click Me'),
),
],
),
),
);
}
}
Video
Disabled ElevatedButton
If the onPressed or onLongPress callbacks are null, then the ElevatedButton will be displayed as a disabled button.
In the following Flutter Application, we shall display an ElevatedButton with neither onPressed nor onLongPress callbacks set.
main.dart
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
/// main application widget
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Tutorial';
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: _title,
home: MyStatelessWidget(),
);
}
}
/// stateless widget that the main application instantiates
class MyStatelessWidget extends StatelessWidget {
const MyStatelessWidget({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('ElevatedButton Tutorial')),
body: Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
ElevatedButton(
onPressed: null,
child: const Text('Click Me'),
),
],
),
),
);
}
}
Screenshot
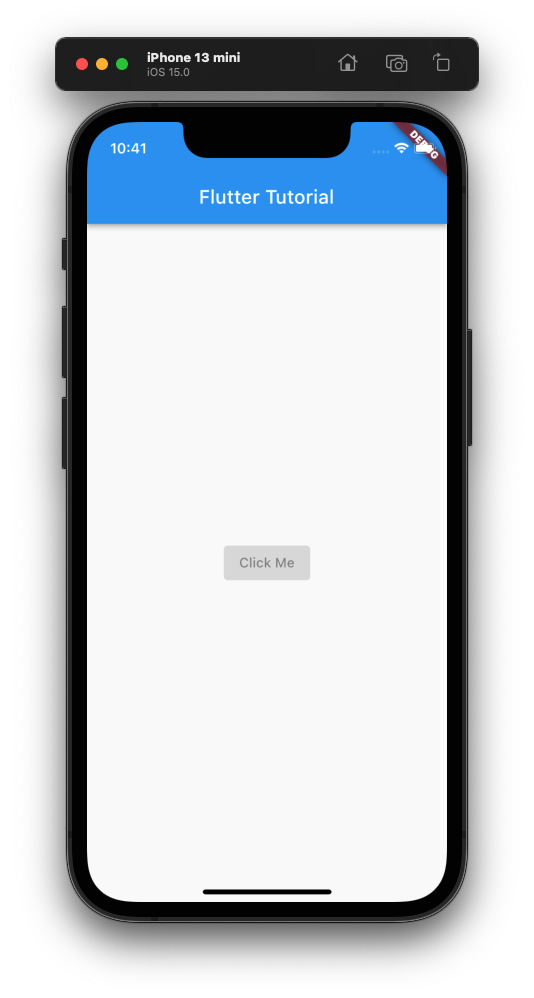
Conclusion
In this Flutter Tutorial, we learned how to display an ElevatedButton in our Flutter Application, with examples.