Flutter Text Widget
Flutter Text widget allows you to display text in your Flutter application.
In this tutorial, we will learn how to use a Text Widget in your application. Also, we will briefly touch on how to style a text widget with references to detailed tutorials.
Example: Flutter Text Widget
In this example, we will create a Flutter application, and use Text Widget to display title in application bar and a message in the body of an application.
Step 1
Create a Flutter application from any of your favorite IDE. This is a good old counter example.
Step 2
Replace the contents of lib/main.dart
with the following code.
main.dart
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Tutorial',
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Text Widget Tutorial'),
),
body: Center(
child: Text('Hello World'),
),
),
);
}
}
In this application, we are using a Scaffold Widget wrapped in MaterialApp. In the appBar and body of Scaffold, we shall use Text widget to display title and body respectively.
This is the simple scenario of using a Text widget, just passing the string you want to display, to Text() class.
When you run this application, the text widgets are displayed in the application as shown in the following figure.
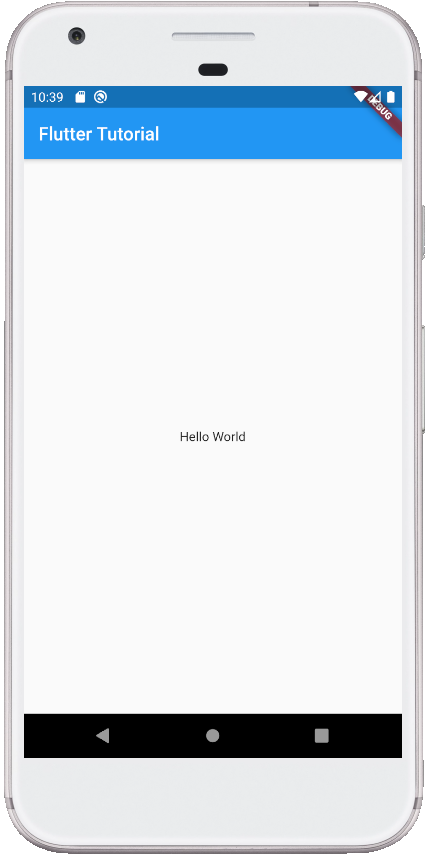
Step 3
Let us change the text provided to text widgets and hot reload the application.
main.dart
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Tutorial',
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Text Widget Tutorial'),
),
body: Center(
child: Text('Hello World! This is a text widget.'),
),
),
);
}
}
Screenshot
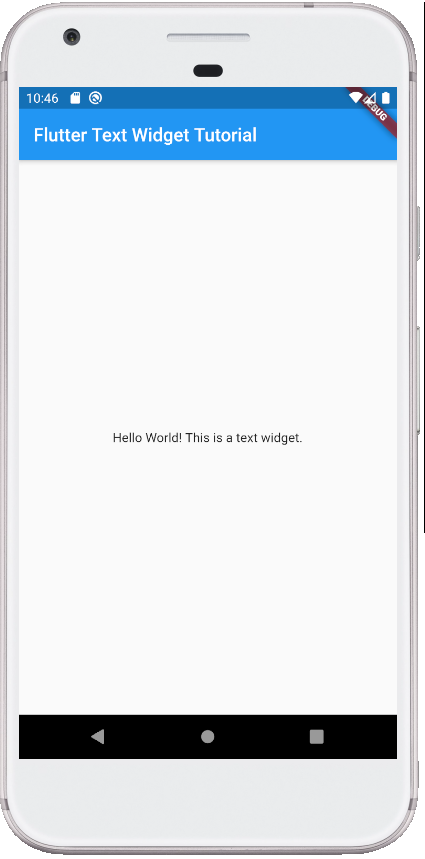
Text Widget Styling
You can style a text widget in Flutter.
Following are some of the scenarios where we try to style a Text Widget.