Flutter WebView
Flutter WebView widget displays a browser like space to show the webpage specified by URL. So, you can display a webpage just like another widget in your mobile application.
In this tutorial, we will learn how to use WebView widget in Android Application.
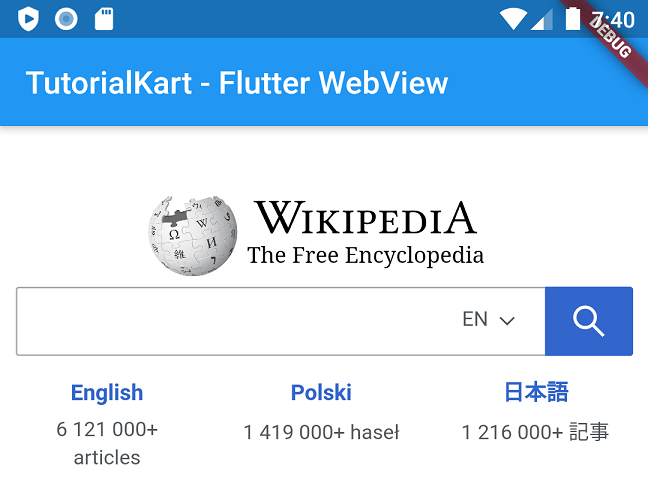
Following is a step by step process to use WebView widget in your application.
Step 1 – pubspec.yaml dependencies
Open pubspec.yaml file, and under dependencies add webview_flutter as shown below.
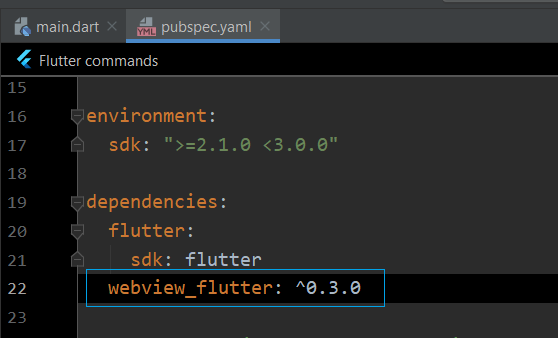

Step 2: Import webview package
Now, you can import webview package in your dart file.
import 'package:webview_flutter/webview_flutter.dart';
Step 3: Use WebView widget
Now you can use the WebView widget in your application.
WebViewController _controller; ... WebView( initialUrl: 'https://www.tutorialkart.com/', javascriptMode: JavascriptMode.unrestricted, onWebViewCreated: (WebViewController webViewController) { _controller = webViewController; }, ),
Example Application
In the following example, we have built a Flutter Application with a WebView widget.
To recreate this example, create a Flutter Application, follow the above steps and replace the main.dart with the following.
main.dart
import 'package:flutter/material.dart'; import 'package:webview_flutter/webview_flutter.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { WebViewController _controller; // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: Scaffold( appBar: AppBar( title: Text("TutorialKart - Flutter WebView"), ), body: Center( child: WebView( initialUrl: 'https://www.tutorialkart.com/', javascriptMode: JavascriptMode.unrestricted, onWebViewCreated: (WebViewController webViewController) { _controller = webViewController; }, ), ), ), ); } }
Following is the pubspec.yaml file. Observe the dependencies section.
pubspec.yaml
name: flutter_webview description: An example Flutter application to demonstrate WebView widget. version: 1.0.0+1 environment: sdk: ">=2.1.0 <3.0.0" dependencies: flutter: sdk: flutter webview_flutter: ^0.3.0 cupertino_icons: ^0.1.2 dev_dependencies: flutter_test: sdk: flutter flutter: uses-material-design: true
Run the application on an Emulator or a physical device, and you should get the following output.
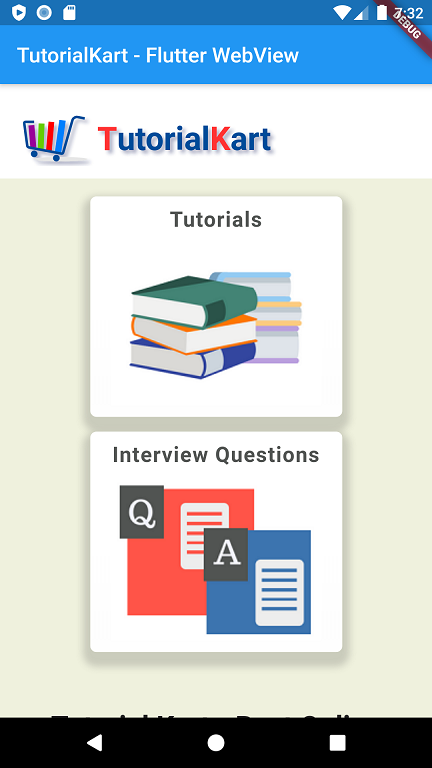
Conclusion
In this Flutter Tutorial, we learned how to create a WebView widget in your Flutter Application.