Flutter – Center Widget
Flutter Center widget center aligns its child widget within itself, along vertical and horizontal axes.
Syntax
The syntax of a Center widget to center other widget is
Center( child: someWidget, )
ADVERTISEMENT
Example
In the following example, we create a Flutter Application with a Text widget wrapped in a Center widget.
main.dart
import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Tutorial'; @override Widget build(BuildContext context) { return MaterialApp( title: _title, home: Scaffold( appBar: AppBar(title: const Text(_title)), body: const MyStatefulWidget(), ), ); } } class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { @override Widget build(BuildContext context) { return const Center( child: Text('Hello World', style: TextStyle(fontSize: 30),), ); } }
Screenshot – iPhone Simulator
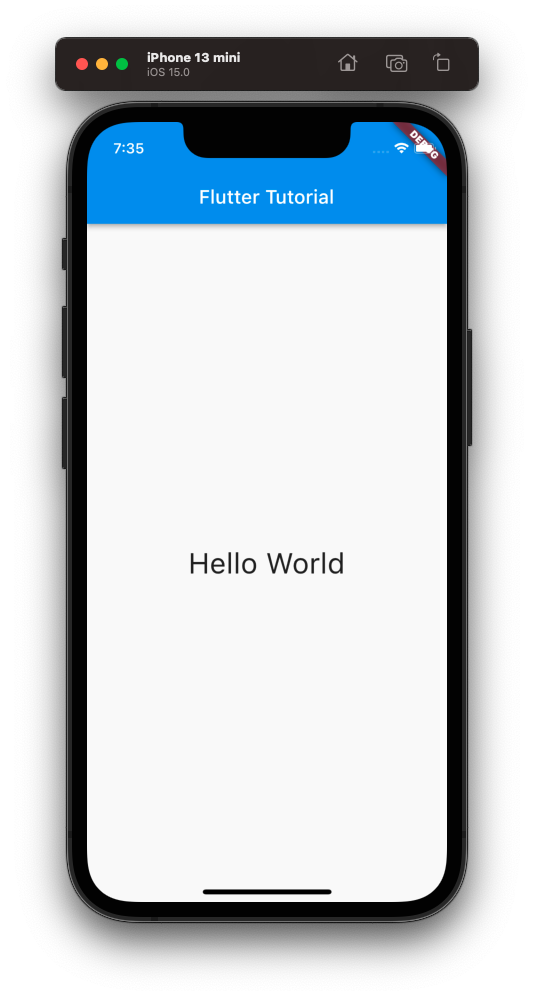
Screenshot – Android Emulator
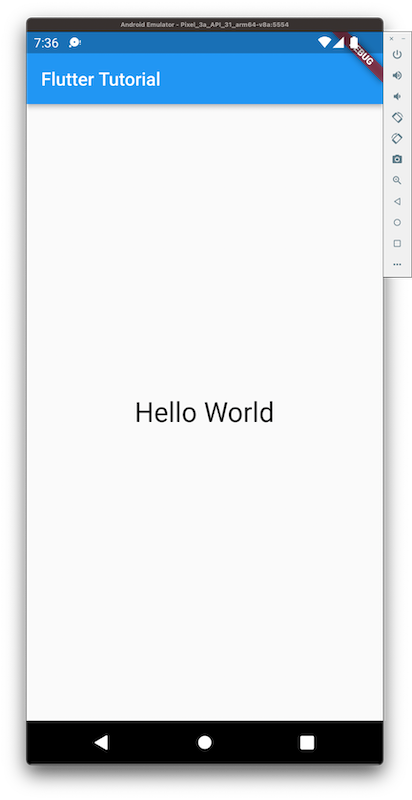
Text Widget without Center Widget
If there is no Center widget around Text widget, then the Text widget would appear as shown in the following.
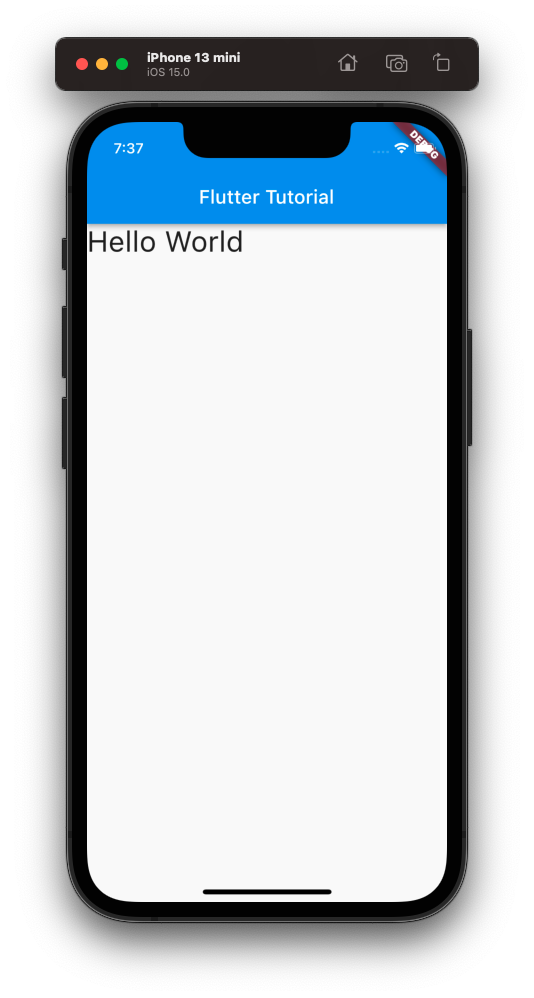
Conclusion
In this Flutter Tutorial, we learned what Center widget is, and how to use it in our Flutter application, with examples.