Center Align Title of Flutter Application
Usually, we use a Text Widget to display title of a Flutter Application.
And sometimes, based on the design requirements or some other situations, you may need to align the title of your application to center.
In this tutorial, we will align the title of a Flutter Application to center.
To center align the title, just wrap the title Text widget inside a Center widget as shown below.
</>
Copy
title: Center(
child: Text('Flutter Tutorial'),
)
Example – Center Align Title
In this example, we will create a basic Flutter Hello World application and center align the title using Center widget.
lib/main.dart
</>
Copy
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Tutorial',
home: Scaffold(
appBar: AppBar(
title: Center(
child: Text('Flutter Tutorial'),
),
),
body: Center(
child: Text('Hello World!'),
),
),
);
}
}
Screenshot
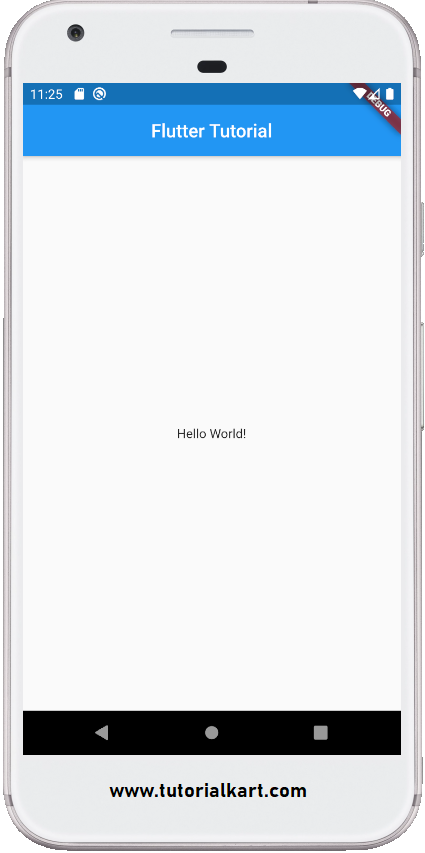
Conclusion
In this Flutter Tutorial, we learned how to center align title of your Flutter Application using Center widget.