Change Font Size of Text Widget
You can change the font size of text in a Text Widget using style property.
Create a TextStyle
object with fontSize
and specify this object as style
for Text Widget.
A quick code snippet is shown below.
</>
Copy
Text(
'Hello World!',
style: TextStyle(fontSize: 25),
),
Change the value for fontSize
to change the font size of text in Text Widget.
Example – Change Font Size of Text in Text Widget
Following is a simple example, where we change the font size of text in Text Widget.
lib\main.dart
</>
Copy
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Tutorial',
home: Scaffold(
appBar: AppBar(
title: Text('Flutter Tutorial'),
),
body: Center(
child: Text(
'Hello World! This is a text widget.',
style: TextStyle(fontSize: 25),
),
),
),
);
}
}
Screenshot
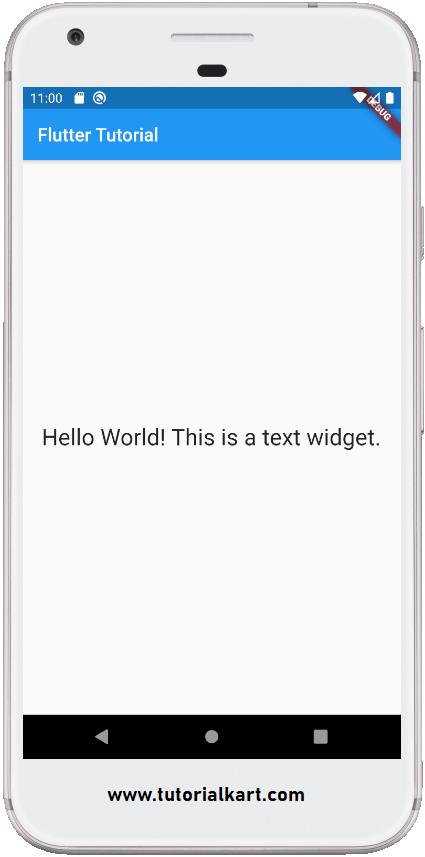
Now, change the font size to 40 and observe the changes in your application. It should be something like as shown in the folowing screenshot.
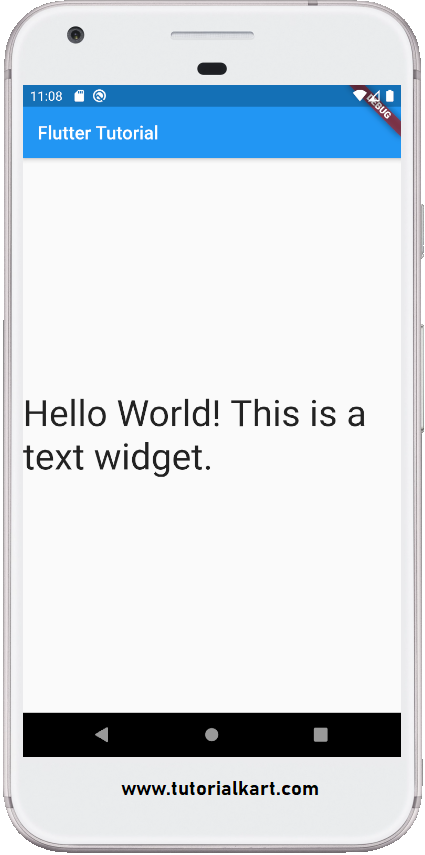
Conclusion
In this Flutter Tutorial, we learned how to change the font size of a Text Widget using TextStyle class.