Flutter Column
Flutter Column widget is used to display its widgets in a vertical array.
In this tutorial, we will get introduced to Column class, and how to use it to display a Column layout in our Application.
Syntax
The syntax to display a Column widget with children is shown in the following.
Column( children: const <Widget>[ //some widgets ], )
ADVERTISEMENT
Example
In the following Flutter Application, we shall display a Column widget with four children widgets of which two are Text widgets and two are Icon widgets. We shall wrap the Column widget in a Center widget to display the column at the centre of the screen along horizontal axis.
main.dart
import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); /// main application widget. class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Tutorial'; @override Widget build(BuildContext context) { return const MaterialApp( title: _title, home: MyStatefulWidget(), ); } } /// stateful widget that the main application instantiates class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } /// private State class that goes with MyStatefulWidget class _MyStatefulWidgetState extends State<MyStatefulWidget> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Column Widget Tutorial'), ), body: Center( child: Column( children: const <Widget>[ Text('Text 1', style: TextStyle(fontSize: 24.0),), Text('Text 2', style: TextStyle(fontSize: 24.0),), Icon( Icons.beach_access, color: Colors.pink, size: 30.0, ), Icon( Icons.audiotrack, color: Colors.green, size: 30.0, ), ], ) ), ); } }
Screenshot – Android Emulator
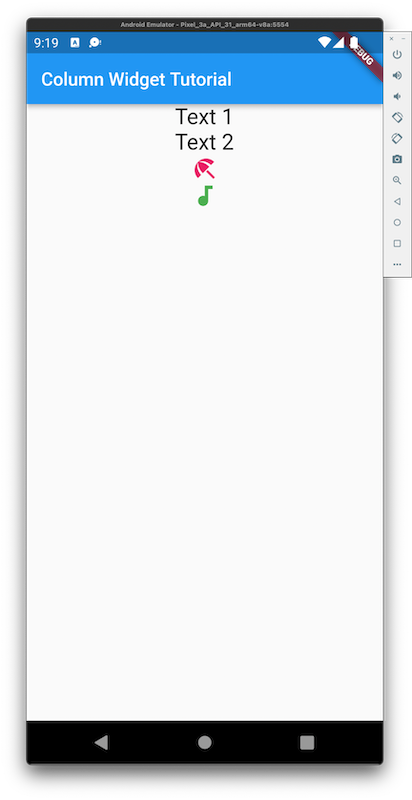
Screenshot – iPhone Simulator
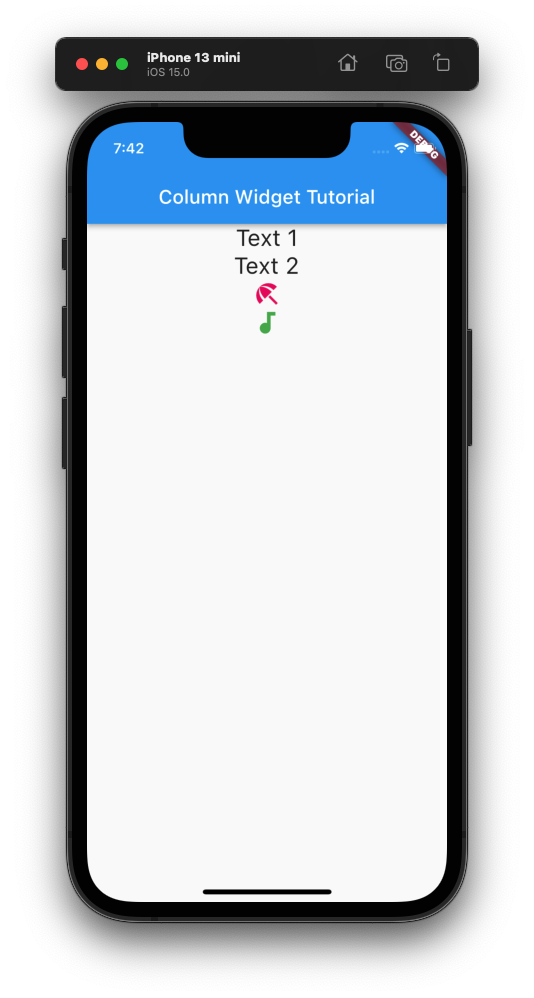
Conclusion
In this Flutter Tutorial, we learned how to display a Column widget in our Flutter Application, with examples.