Flutter Scaffold
Flutter Scaffold is used to display a basic material design layout that contains application bar, body, bottom navigation bar, bottom sheet, drawer, floating action button, persistent footer buttons, etc.
In this tutorial, we will get introduced to Scaffold class, and how to use it to display a Scaffold layout in our Application.
Syntax
The syntax to display a Scaffold layout with app bar, body, and floating action button, is shown in the following.
Scaffold( appBar: /*some application bar*/, body: /*some body*/, floatingActionButton: /*some floating action button*/, );
Example
In the following Flutter Application, we shall display a Scaffold with an appBar, body, and a floating action button.
In build() method of _MyStatefulWidgetState class, we are returning a Scaffold.
main.dart
import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); /// main application widget. class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Tutorial'; @override Widget build(BuildContext context) { return const MaterialApp( title: _title, home: MyStatefulWidget(), ); } } /// stateful widget that the main application instantiates class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } /// private State class that goes with MyStatefulWidget class _MyStatefulWidgetState extends State<MyStatefulWidget> { int _count = 0; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Scaffold Tutorial'), ), body: Center(child: Text('You have pressed the button $_count times.')), floatingActionButton: FloatingActionButton( onPressed: () => setState(() => _count++), tooltip: 'Increment Counter', child: const Icon(Icons.add), ), ); } }
Screenshot – Android Emulator
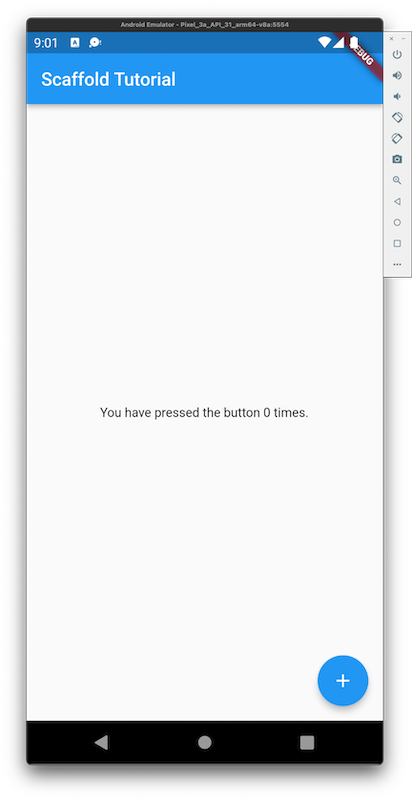
Screenshot – iPhone Simulator
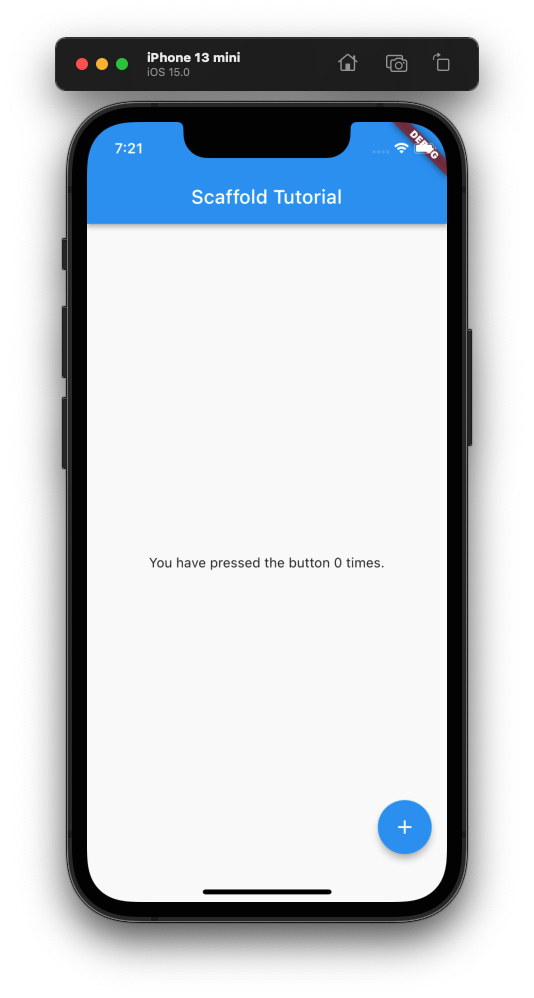
Conclusion
In this Flutter Tutorial, we learned how to display a Scaffold layout in our Flutter Application, with examples.