Flutter ListView
Flutter ListView widget displays a set of items in a scrollable list. Pass the list of items to the children property of ListView widget, and you have a ListView in your Flutter application.
You can make the ListView scroll in either of the directions: horizontal, vertical; using scrollDirection property. Or you can also make it never scroll using physics property set to NeverScrollableScrollPhysics() object.
In this tutorial, we will create a basic ListView widget that displays a set of items.
Syntax
The syntax of ListView widget with children
property is
ListView(
children: <Widget>[
//widget items
],
)
Example
In this example, we will use ListView to display widgets as a list that is scrollable vertically.
Create a new Flutter project and use the following main.dart file in your application.
main.dart
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(
title: Text('TutorialKart - Flutter ListView'),
),
body: ListView(
children: <Widget>[
Container(
height: 50,
color: Colors.lime[800],
child: const Center(child: Text('Apple')),
),
Container(
height: 50,
color: Colors.lime[600],
child: const Center(child: Text('Banana')),
),
Container(
height: 50,
color: Colors.lime[400],
child: const Center(child: Text('Mango')),
),
Container(
height: 50,
color: Colors.lime[200],
child: const Center(child: Text('Orange')),
),
],
),
),
);
}
}
Run the application and you would get the ListView displayed in the application as shown below.
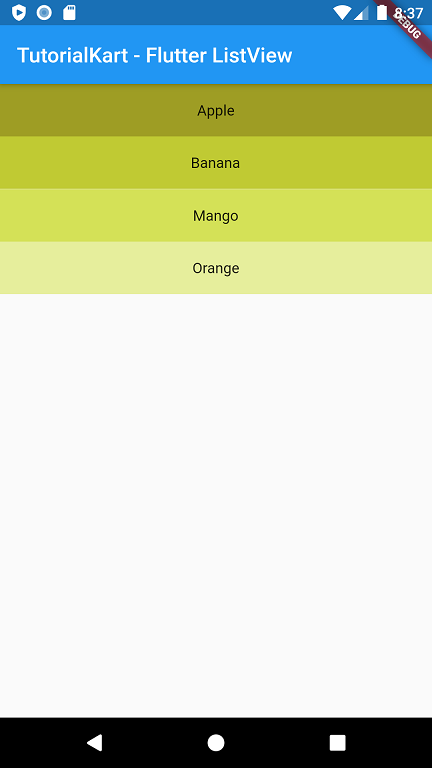
Conclusion
In this Flutter Tutorial, we learned how to create a ListView widget in Flutter Application.