Flutter Canvas Draw Rectangle
You can draw custom shapes in your application. But, let us start slow and steady. Let us start with drawing a rectangle.
In this tutorial, we shall learn how to draw a rectangle on a Canvas using CustomPainter widget.
Function to Draw Rectangle – Canvas.drawRect()
Following is the syntax of drawRect() function in Flutter.
void drawRect(Rect rect, Paint paint)
A quick example of drawRect() is given below.
canvas.drawRect(Offset(100, 100) & const Size(200, 150), Paint());
Of course you can change the offset, size of rectangle and paint properties.
Flutter Canvas – Draw Rectangle
To draw a rectangle in our Flutter Application, we shall follow the below steps.
- Create a class that extends CustomPainter. This is our widget that has canvas and allows user to paint on to the canvas.
- Override paint() and shouldRepaint() methods of the CustomPainter class. In the paint() method, we have access to canvas and size of the canvas.
- Draw a rectangle on the canvas using canvas.drawRect() method.
main.dart
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter TutorialKart',
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter - www.tutorialkart.com'),
),
body: ListView(children: <Widget>[
Container(
width: 400,
height: 400,
child: CustomPaint(
painter: OpenPainter(),
),
),
]),
);
}
}
class OpenPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint1 = Paint()
..color = Color(0xff995588)
..style = PaintingStyle.fill;
canvas.drawRect(Offset(100, 100) & const Size(200, 150), paint1);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
Run the application in your Android Device or Android Emulator.
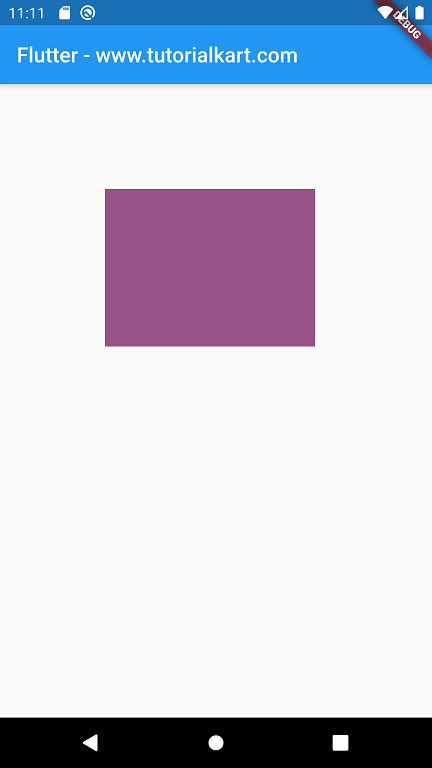
Flutter Canvas – Update Rectangle
You can update any property of the rectangle you would like to change in runtime.
To change a property of the rectangle you are painting, follow these steps.
Step 1: Declare the properties you would like to change, as a variable. Assign the default or initial value to it.
Color rectColor = Color(0xff000000);
Size rectSize = Size(200, 150);
Step 2: Use this variables while you are painting the rectangle in CustomPainter class.
void paint(Canvas canvas, Size size) {
var paint1 = Paint()
..color = rectColor
..style = PaintingStyle.fill;
canvas.drawRect(Offset(100, 100) & rectSize, paint1);
}
Step 3: When a change has to be made, like when user presses a button, assign the new value for property using the variable(declared in step 1).
rectColor = Color(0xff885599);
rectSize = Size(200, 250);
Following is the complete code of this example application where we are changing the color and size of a rectangle.
main.dart
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
Color rectColor = Color(0xff000000);
Size rectSize = Size(200, 150);
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter TutorialKart',
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter - www.tutorialkart.com'),
),
body: ListView(children: <Widget>[
Text(
'My Canvas',
textAlign: TextAlign.center,
style: TextStyle(fontSize: 20),
),
Container(
width: 400,
height: 400,
child: CustomPaint(
painter: OpenPainter(),
),
),
RaisedButton(
child: Text('Repaint Canvas'),
onPressed: (){
setState(() {
rectColor = Color(0xff885599);
rectSize = Size(200, 250);
});
},
),
]),
);
}
}
class OpenPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint1 = Paint()
..color = rectColor
..style = PaintingStyle.fill;
canvas.drawRect(Offset(100, 100) & rectSize, paint1);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
Run the application in your Android Smartphone or Emulator, you will get the left screen as shown in the following screenshot. When you click the Repaint Canvas
button, the color the rectangle is changed to a new value.
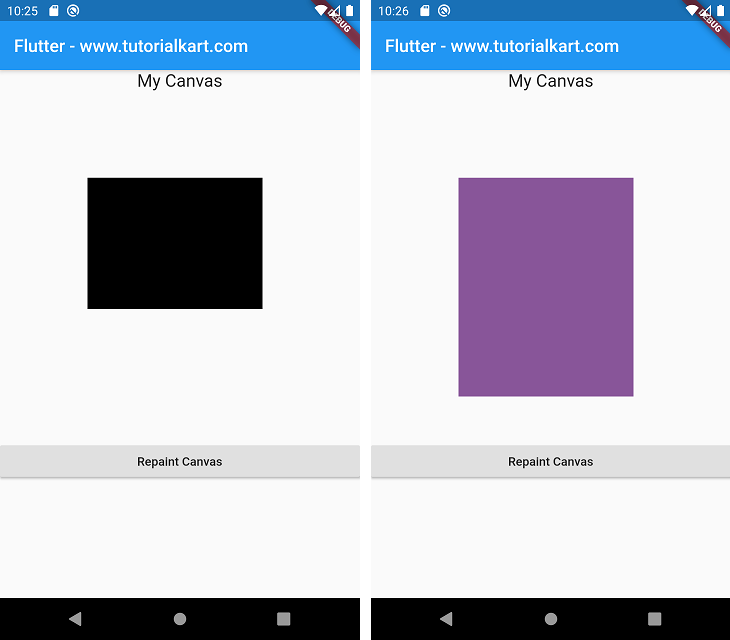
Conclusion
In this Flutter Tutorial, we learned how to draw a rectangle on a Canvas using CustomPainter class.