Flutter Image Height
To set specific height for Image widget in Flutter Application, set height property of Image
object with required double value.
Syntax
The syntax to set height property for Image widget is
const Image( height: 200, )
ADVERTISEMENT
Example
In the following example, we create a Flutter Application with two Image widgets. The height of first Image widget is set to 100, and the height of second Image widget is set to 200.
main.dart
import 'dart:ui'; import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Tutorial'; @override Widget build(BuildContext context) { return MaterialApp( title: _title, home: Scaffold( appBar: AppBar(title: const Text(_title)), body: const MyStatefulWidget(), ), ); } } class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { @override Widget build(BuildContext context) { return Center( child: Column( children: <Widget>[ Container( margin: const EdgeInsets.all(20), child: const Image( height: 100, image: NetworkImage( 'https://www.tutorialkart.com/img/lion.jpg'), ), ), Container( margin: const EdgeInsets.all(20), child: const Image( height: 200, image: NetworkImage( 'https://www.tutorialkart.com/img/lion.jpg'), ), ), ], ), ); } }
Screenshot – iPhone Simulator
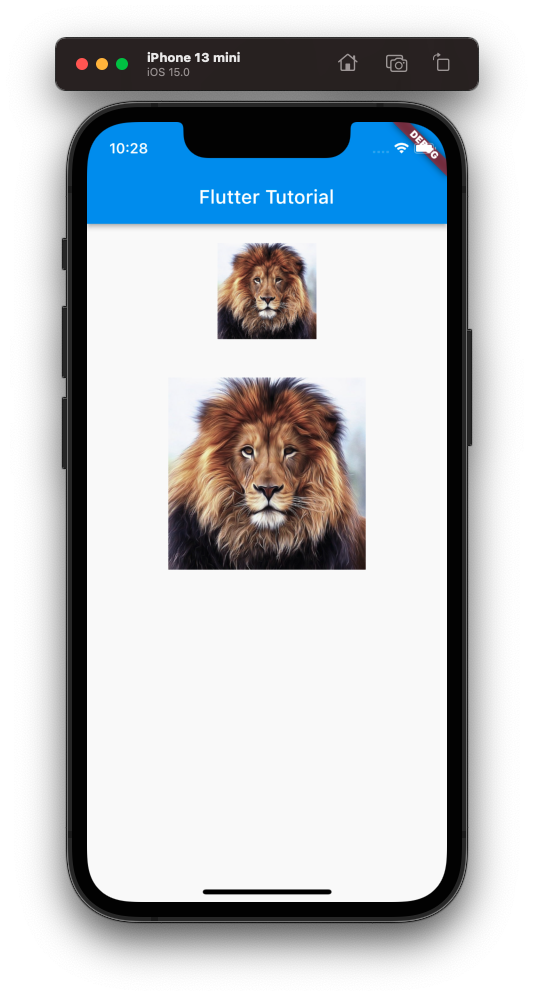
Screenshot – Android Emulator
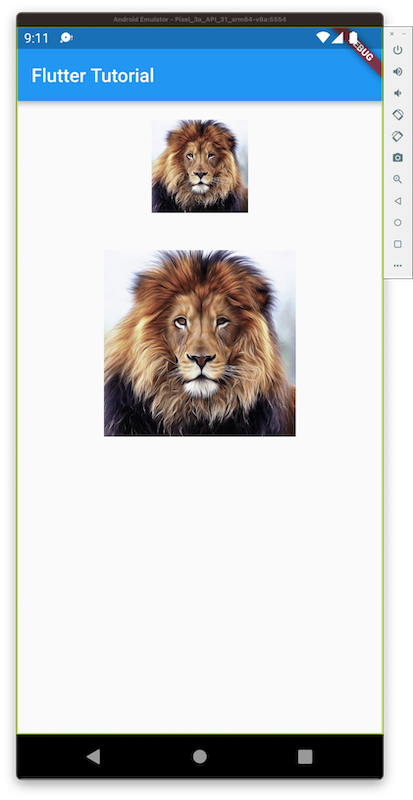
Conclusion
In this Flutter Tutorial, we learned how to set specific height for an Image widget, with examples.