Flutter RaisedButton
In Flutter, RaisedButton widget is a material design concept of a button with elevation. It provides dimensionality to your UI along z-axis with clues of shadow.
RaisedButton is depreciated. Please refer ElevatedButton to display a button.
You can set many properties of RaisedButton like text color, button color, color of button when disabled, animation time, shape, elevation, padding, etc.
You can also disable the button using enabled property.
There are callback functions:
- onPressed() is triggered when user presses this button.
- onLongPress() is triggered when user long presses on this button.
Please note that Raised button shall be in disabled state if both onPressed() and onLongPress() callbacks are not provided. In this state, any properties applied to the button are not effective.
Example – Flutter RaisedButton
In this example, we shall build a Flutter Application with many raised buttons. Each of them shall demonstrate a property of RaisedButton. Only some of the properties are discussed here. You may explore RaisedButton documentation to discover all of its properties.
main.dart
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter RaisedButton - tutorialkart.com'),
),
body: Center(
child: Column(children: <Widget>[
Text(
'Raised Buttons with Different Properties',
style: TextStyle(fontSize: 16),
),
RaisedButton(child: Text('Disabled Button')),
RaisedButton(
child: Text('Default Enabled'),
onPressed: () {},
),
RaisedButton(
child: Text('Text Color Changed'),
textColor: Colors.red,
onPressed: () {},
),
RaisedButton(
child: Text('Color Changed'),
color: Colors.green,
onPressed: () {},
),
RaisedButton(
child: Text('Button with Padding'),
padding: EdgeInsets.all(20),
onPressed: () {},
),
RaisedButton(
child: Text('More Rounded Corners'),
color: Colors.purpleAccent,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(16.0))),
onPressed: () {},
),
RaisedButton(
child: Text('Elevation increased'),
elevation: 5,
onPressed: () {},
),
RaisedButton(
child: Text('Splash Color as red'),
splashColor: Colors.red,
onPressed: () {},
),
RaisedButton(
child: Text('Zero Elevation'),
elevation: 0,
onPressed: () {},
),
RaisedButton(
onPressed: () {},
textColor: Colors.white,
padding: const EdgeInsets.all(0.0),
child: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
colors: <Color>[
Color(0xFF0D47A1),
Color(0xFF1976D2),
Color(0xFF42A5F5),
],
),
),
padding: const EdgeInsets.all(10.0),
child: const Text('Gradient Color'),
),
),
]))),
);
}
}
Run this application, and you should see UI similar to the following screenshot.
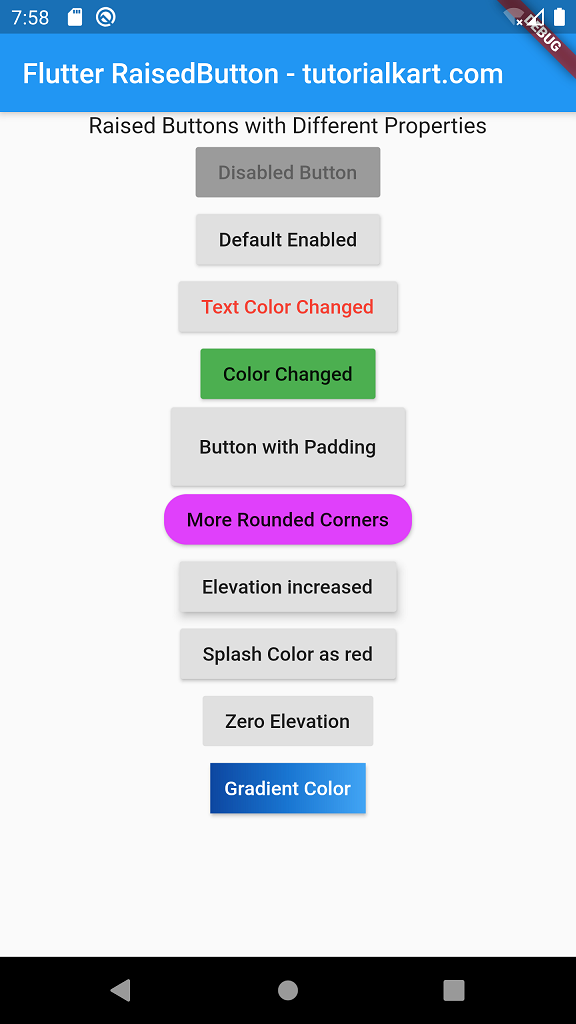
Conclusion
In this Flutter Tutorial, we learned how to use RaisedButton in Flutter Application with specific values set to different properties.