Flutter – Container Box Shadow
To set box shadow for Container widget, set its decoration
property with a BoxDecoration object. Inside BoxDecoration, set boxShadow
property with required array of BoxShadow objects.
Sample Code
A quick code snippet to set box shadow for Container widget with specific colors, offset, and radii is
</>
Copy
Container(
decoration: BoxDecoration(
boxShadow: const [
BoxShadow(
color: Colors.grey,
blurRadius: 5,
spreadRadius: 1,
offset: Offset(4, 4)
),
],
),
)
Example
In the following example, we create a Flutter Application with a Container widget, and set its box shadow with color of grey, blur radius of 5, spread radius of 1, and an offset of (4, 4).
main.dart
</>
Copy
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Tutorial';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
@override
Widget build(BuildContext context) {
return Center(
child: Column(
children: <Widget>[
const SizedBox(height: 10,),
Container(
height: 200,
width: 200,
alignment: Alignment.center,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(10),
boxShadow: const [
BoxShadow(
color: Colors.grey,
blurRadius: 5,
spreadRadius: 1,
offset: Offset(4, 4)
),
],
color: Colors.green[200],
),
child: const Text('Container 1'),
),
]
),
);
}
}
Screenshot – iPhone Simulator
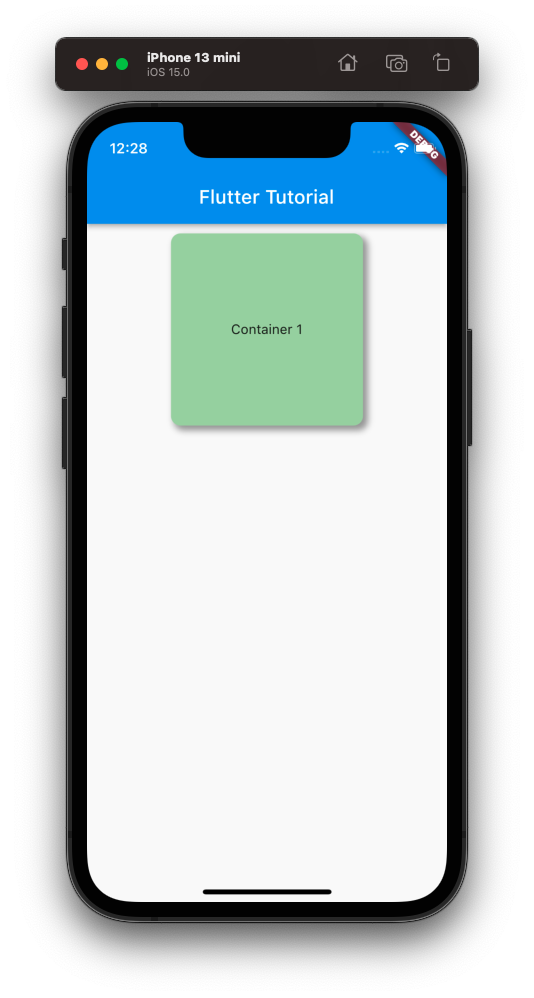
Screenshot – Android Emulator
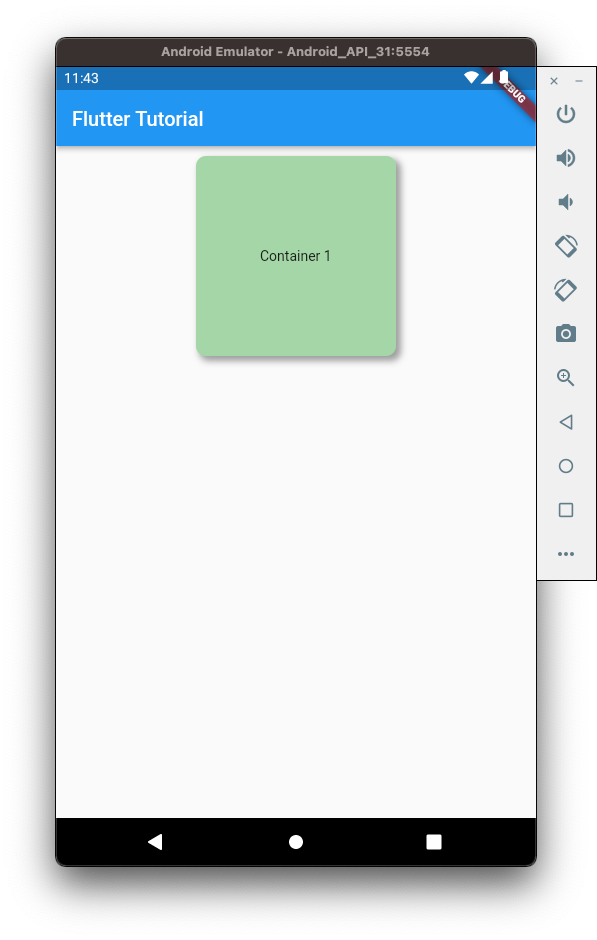
Conclusion
In this Flutter Tutorial, we learned how to set box shadow for Container widget, with examples.