Flutter – Banner Widget
Flutter Banner widget displays a message box diagonally on the corner of its child widget.
Syntax
The syntax of a Center widget to center other widget is
</>
Copy
Banner(
message: 'New Arrival',
location: BannerLocation.topStart,
child: someWidget,
)
The message to be displayed in the banner is specified via message
property, and the location of the banner on the child widget is specified via location
property.
Example
In the following example, we create a Flutter Application with a Banner widget. Banner widget’s child is a Container widget with Text. We shall display the banner on the top left of the the child Container.
main.dart
</>
Copy
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Tutorial';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
@override
Widget build(BuildContext context) {
return Center(
child: Container(
padding: const EdgeInsets.all(10),
child: Banner(
message: 'New Arrival',
location: BannerLocation.topStart,
child: Container(
height: 200,
width: 200,
color: Colors.yellow,
alignment: Alignment.center,
child: const Text('Some Item'),
),
),
)
);
}
}
Screenshot – iPhone Simulator
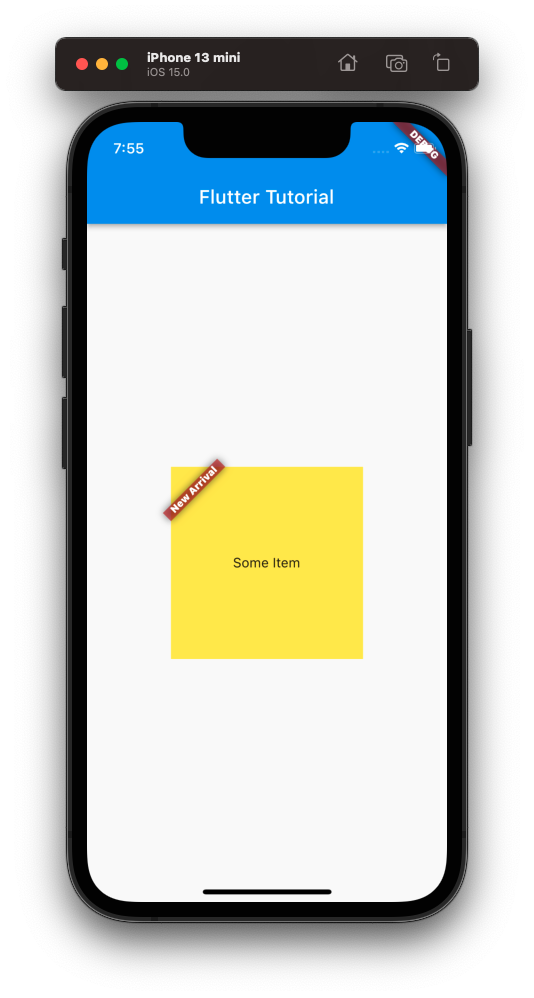
Screenshot – Android Emulator
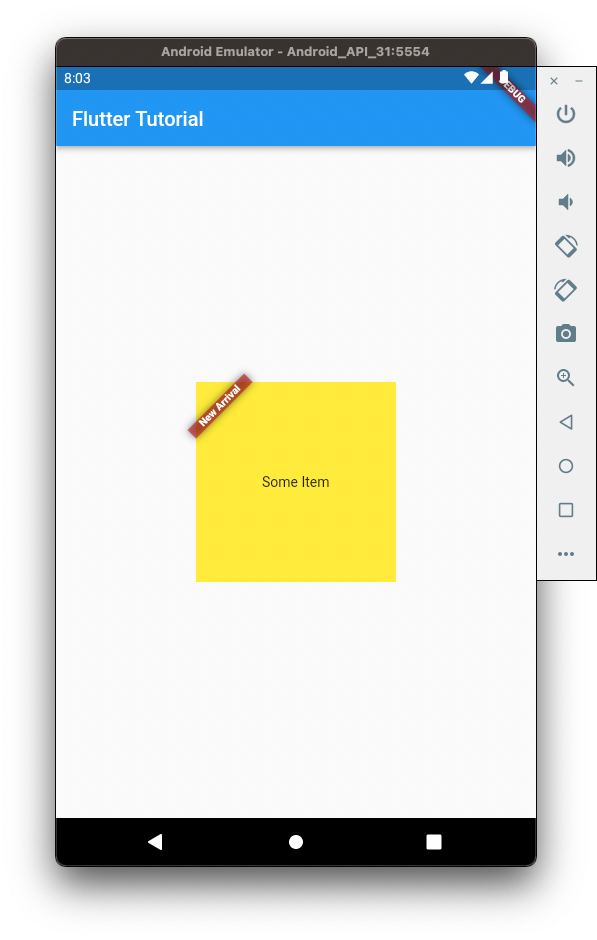
Conclusion
In this Flutter Tutorial, we learned what Banner widget is, and how to use it in our Flutter application, with examples.