Flutter – Container Margin
To set Margin for Container widget in Flutter, set margin
property wit the required EdgeInsets value. We can set margin for top, right, bottom, and left with separate values using EdgeInsets.fromLTRB(), or a set a same value for margin on all sides.
Syntax
The following is an example code snippet to set same margin for all sides of the Container widget.
Container( margin: const EdgeInsets.all(20), )
The following is an example code snippet to set margin for Container widget, with specific values for respective sides.
Container( margin: const EdgeInsets.fromLTRB(20, 10, 20, 10), )
Example
In the following example, we create a Flutter Application with three Container widgets. Each of the Container has an outer Container widget to demonstrate the margin of the inner Container widget.
- No margin is set for the first Container widget.
- Margin of 40 is set for all the sides of the second Container widget.
- Margin of 60, 50, 20, and 40 is set for the left, top, right, and bottom sides respectively for the third Container widget.
main.dart
import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Tutorial'; @override Widget build(BuildContext context) { return MaterialApp( title: _title, home: Scaffold( appBar: AppBar(title: const Text(_title)), body: const MyStatefulWidget(), ), ); } } class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { @override Widget build(BuildContext context) { return Center( child: Column( children: <Widget>[ const SizedBox(height: 10,), Container( color: Colors.red[100], child: Container( color: Colors.green[200], child: const Text('No Margin'), ), ), const SizedBox(height: 10,), Container( color: Colors.red[100], child: Container( margin: const EdgeInsets.all(40), color: Colors.green[200], child: const Text('Same Margin on all sides'), ), ), const SizedBox(height: 10,), Container( color: Colors.red[100], child: Container( margin: const EdgeInsets.fromLTRB(60, 50, 20, 10), color: Colors.green[200], child: const Text('Different margin on different sides'), ), ), ] ), ); } }
Screenshot – iPhone Simulator
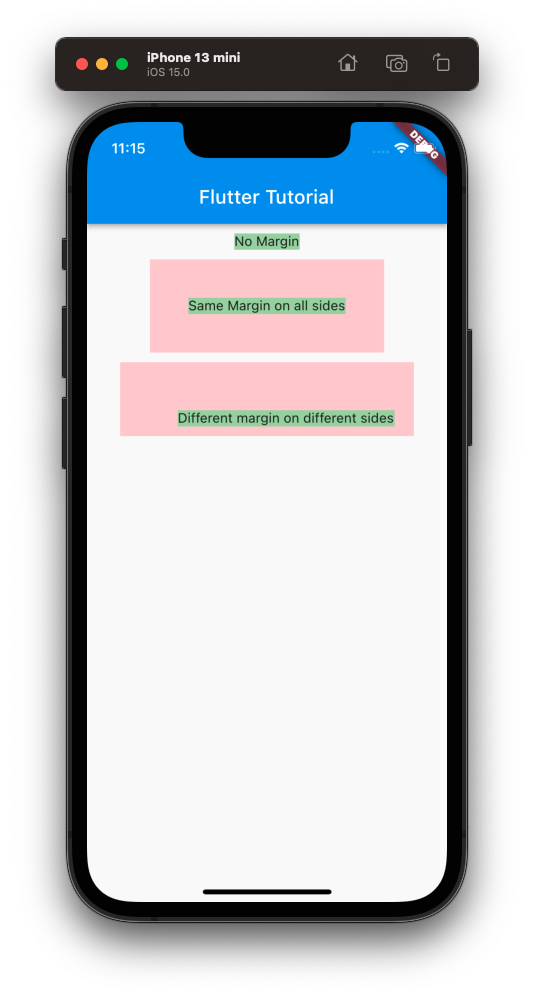
Screenshot – Android Emulator
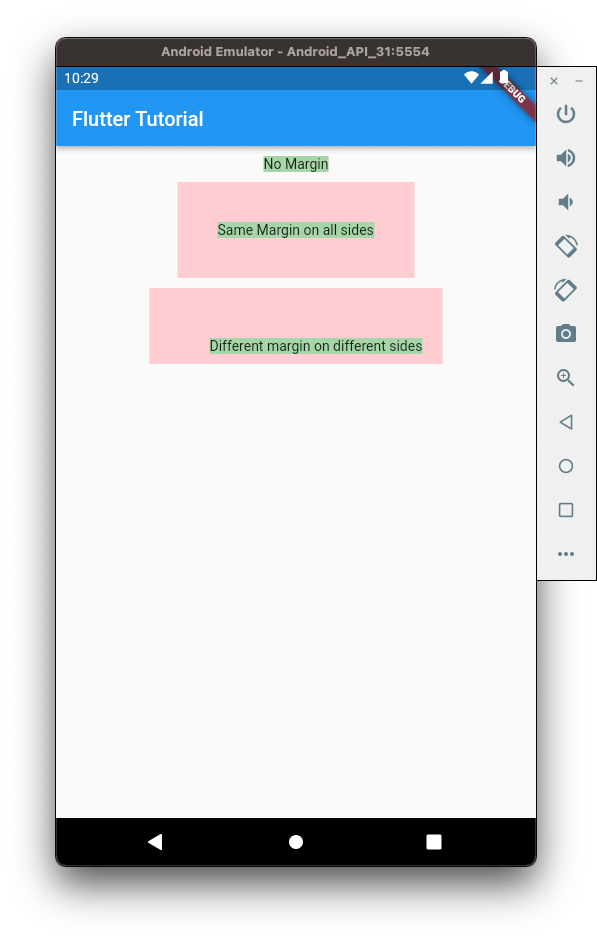
Conclusion
In this Flutter Tutorial, we learned how to set margin for Container widget using margin
property, with examples.