Flutter – GridView Padding
To set padding for GridView in Flutter, set padding property wit the required EdgeInsets value. Via EdgeInsets class object, we can set padding for top, right, bottom, and left with separate values, or a set a same value for padding on all sides.
Code Snippet
The following is an example code snippet to set padding for GridView.
GridView( padding: const EdgeInsets.all(20), )
The following is an example code snippet to set padding for GridView, with specific values for respective sides.
GridView( padding: const EdgeInsets.fromLTRB(20, 10, 20, 10), )
Example
In the following example, we create a Flutter Application with a GridView. We set a padding of 40 for all sides of the GridView.
main.dart
import 'dart:ui'; import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Tutorial'; @override Widget build(BuildContext context) { return MaterialApp( title: _title, home: Scaffold( appBar: AppBar(title: const Text(_title)), body: const MyStatefulWidget(), ), ); } } class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { @override Widget build(BuildContext context) { return GridView.count( primary: false, crossAxisCount: 2, mainAxisSpacing: 10, crossAxisSpacing: 10, padding: const EdgeInsets.all(40), children: <Widget>[ Container( padding: const EdgeInsets.all(8), child: const Text("Tile 1"), color: Colors.orange[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 2"), color: Colors.green[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 3"), color: Colors.red[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 4"), color: Colors.purple[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 5"), color: Colors.blueGrey[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 6"), color: Colors.yellow[200], ), ], ); } }
Screenshot – iPhone Simulator
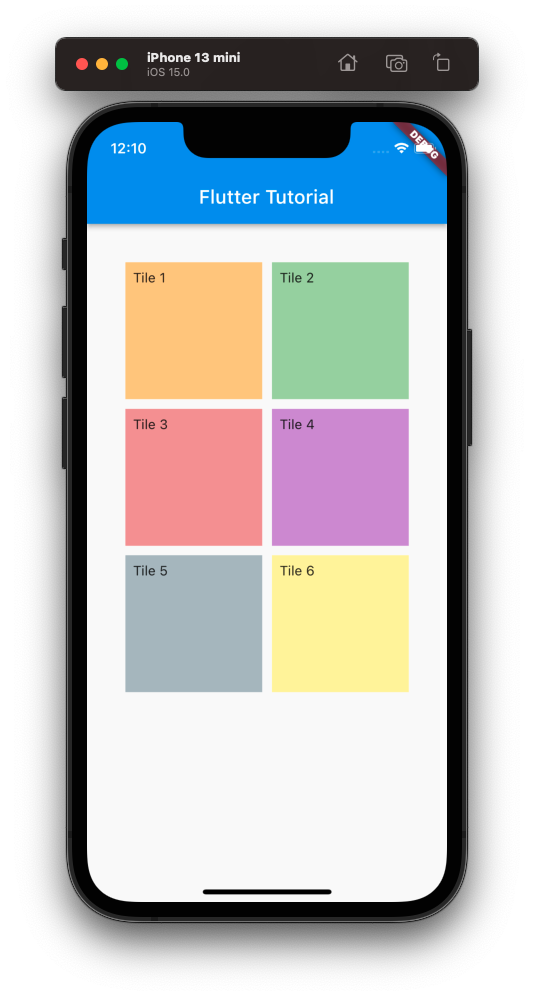
Screenshot – Android Emulator
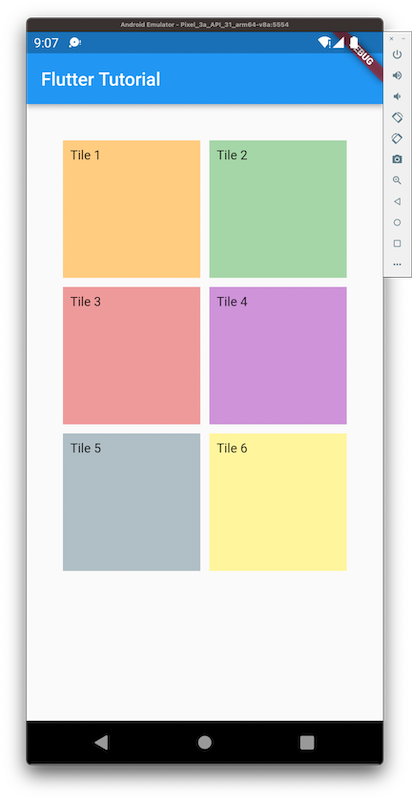
Different Padding for Different Sides
In the following example, we create a Flutter Application with a GridView. We set a padding of 10 for left, 20 for top, 30 for right, and 40 for bottom, using EdgeInsets.fromLTRB() method.
main.dart
import 'dart:ui'; import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Tutorial'; @override Widget build(BuildContext context) { return MaterialApp( title: _title, home: Scaffold( appBar: AppBar(title: const Text(_title)), body: const MyStatefulWidget(), ), ); } } class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { @override Widget build(BuildContext context) { return GridView.count( primary: false, crossAxisCount: 2, mainAxisSpacing: 10, crossAxisSpacing: 10, padding: const EdgeInsets.fromLTRB(10, 20, 30, 40), children: <Widget>[ Container( padding: const EdgeInsets.all(8), child: const Text("Tile 1"), color: Colors.orange[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 2"), color: Colors.green[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 3"), color: Colors.red[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 4"), color: Colors.purple[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 5"), color: Colors.blueGrey[200], ), Container( padding: const EdgeInsets.all(8), child: const Text("Tile 6"), color: Colors.yellow[200], ), ], ); } }
Screenshot
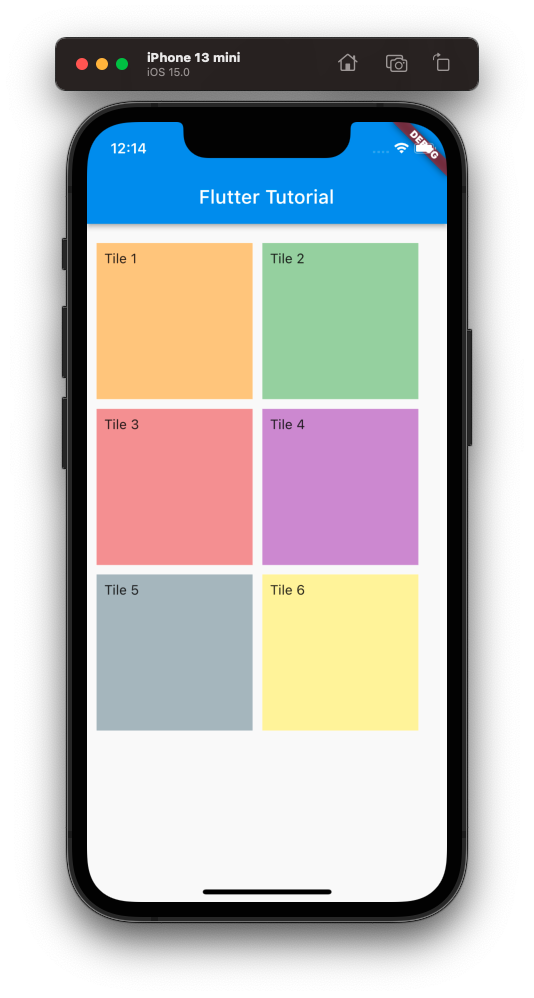
Conclusion
In this Flutter Tutorial, we learned how to set padding for a GridView using padding
property, with examples.