Flutter GridView – Spacing between Items
To set spacing between items along main axis or cross axis, set the required double values for mainAxisSpacing
property and crossAxisSpacing
property respectively.
The default value of mainAxisSpacing
and crossAxisSpacing
is zero. Therefore, if we do not set these values, there would be no space between the items along horizontal and vertical axes.
Code Snippet
A quick code snippet to set spacing for GridView
via mainAxisSpacing and crossAxisSpacing properties is
GridView(
mainAxisSpacing: 10,
crossAxisSpacing: 10,
)
Example
In the following example, we set a spacing of 10 for both main axis spacing and cross axis spacing.
main.dart
import 'dart:ui';
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Tutorial';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
@override
Widget build(BuildContext context) {
return Center(
child: GridView.count(
primary: false,
crossAxisCount: 3,
mainAxisSpacing: 10,
crossAxisSpacing: 10,
padding: const EdgeInsets.all(20),
children: <Widget>[
Container(
padding: const EdgeInsets.all(8),
child: const Text("Tile 1"),
color: Colors.orange[200],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text("Tile 2"),
color: Colors.green[200],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text("Tile 3"),
color: Colors.red[200],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text("Tile 4"),
color: Colors.purple[200],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text("Tile 5"),
color: Colors.blueGrey[200],
),
Container(
padding: const EdgeInsets.all(8),
child: const Text("Tile 6"),
color: Colors.yellow[200],
),
],
)
);
}
}
Screenshot – iPhone Simulator
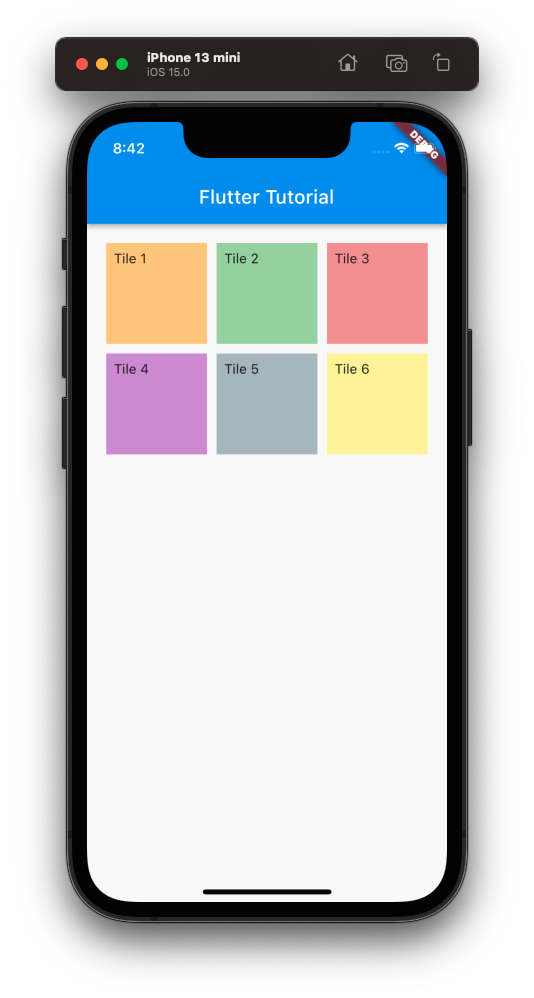
Screenshot – Android Emulator
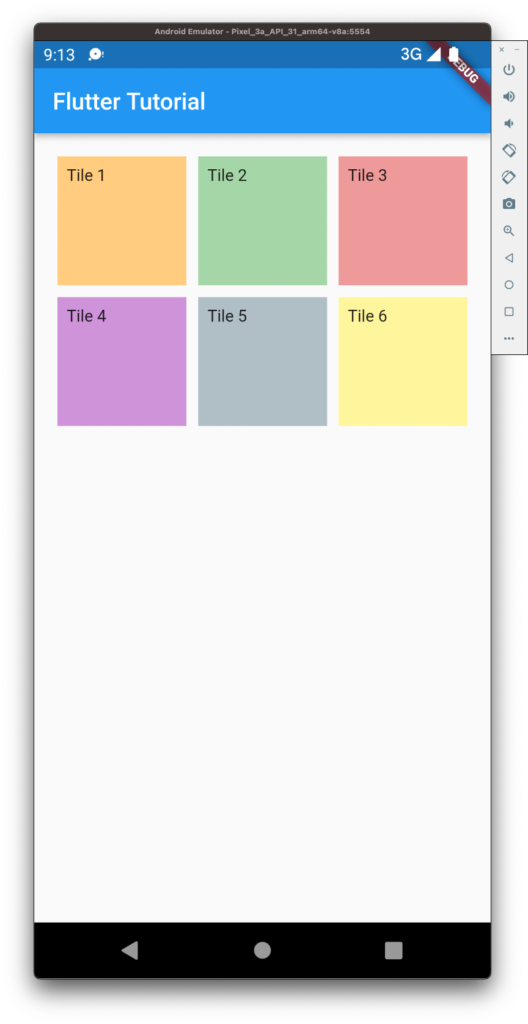
Conclusion
In this Flutter Tutorial, we learned how to set spacing between items of GridView along horizontal and vertical axes (cross and main axes), with examples.