In this PHP tutorial, you shall learn about Two-dimensional Arrays, how to created 2D arrays, access elements of 2D arrays, and traverse the inner arrays of a 2D array, with example programs.
PHP 2D Array
PHP 2D Array is an array with arrays as its elements. In this tutorial, we will learn how to create a 2D array, and access elements of the inner arrays.
Create 2D array
To create a 2D array in PHP, use array() function. The syntax to use array() function to create a 2D array is
$array2D = array( array(value, value, value), array(value, value, value), array(value, value, value) )
You can define as many number of inner arrays as possible in the outer array.
The outer array defines one dimension, and the inner arrays define another dimension. Hence two dimensional array.
In the following example, we will create a 2D array.
PHP Program
<?php $array2D = array( array(7, 8, 9), array(4, 5, 6), array(1, 2, 3) ); ?>
Access Elements in 2D Array
Since the array is two dimensional, we have to provide two indexes. The first index lets you access a specific array in outer array, the second index will let you access the element in the inner array.
In the following example, we will create a 2D array and access element present in the second inner array, and at third position inside this inner array.
PHP Program
<?php $array2D = array( array(7, 8, 9), array(4, 5, 6), array(1, 2, 3) ); $value = $array2D[1][2]; echo $value; ?>
Output
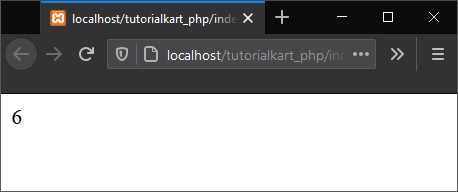
Traverse through 2D Array
We can use nested foreach statement to traverse through all the elements of a given Two-Dimensional array.
In the following example, we will create a two-dimensional array, and print its elements using nested foreach.
PHP Program
<?php $array2D = array( array(7, 8, 9), array(4, 5, 6), array(1, 2, 3) ); foreach ($array2D as $array1D) { foreach ($array1D as $element) { echo $element; echo " "; } echo "<br>"; } ?>
Output
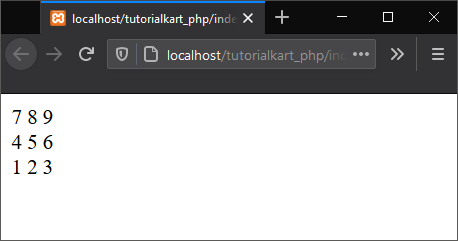
Conclusion
In this PHP Tutorial, we learned how to create and access 2D arrays in PHP, and how to traverse through all the elements using nested foreach.