In this PHP tutorial, you shall learn how to check if given string contains only numeric digits using preg_match() function, with example programs.
PHP – Check if string contains only numeric digits
To check if a string contains only numeric digits in PHP, call preg_match()
function and pass pattern and given string as arguments. The pattern must match one or more numeric digits from starting to end of the string.
Syntax
The syntax to check if string str
contains only numeric digits is
preg_match('/^[0-9]+$/', $str)
The above expression returns a boolean value of true
if the string $str
contains only numeric digits, or false
otherwise.
Examples
1. Positive Scenario – String contains only Numeric Digits
In this example, we take a string in str
such that it contains only numeric digits. For the given value of string, preg_match()
returns true
and if-block executes.
PHP Program
<?php
$str = '123454321';
$pattern = '/^[0-9]+$/';
if ( preg_match($pattern, $str) ) {
echo 'string contains only digits';
} else {
echo 'string does not contain only digits';
}
?>
Output
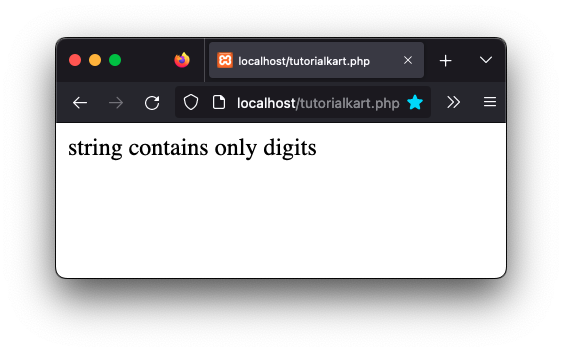
2. Negative Scenario – String contains not only numeric digits
In this example, we take a string in str
such that it contains some alphabets along with numeric digits. For the given value of string, preg_match()
returns false
and else-block executes.
PHP Program
<?php
$str = '1234abc';
$pattern = '/^[0-9]+$/';
if ( preg_match($pattern, $str) ) {
echo 'string contains only digits';
} else {
echo 'string does not contain only digits';
}
?>
Output
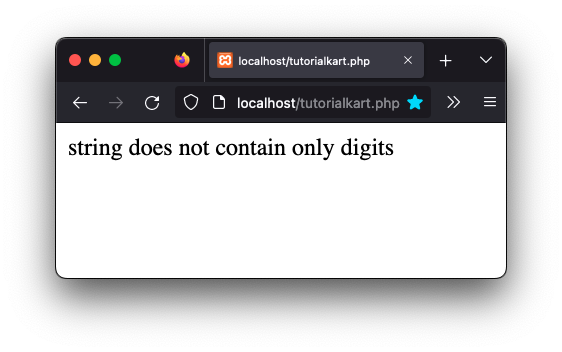
Conclusion
In this PHP Tutorial, we learned how to check if given string contains only numeric digits, using preg_match()
function, with the help of examples.