In this tutorial, you shall learn about switch-case statement in PHP, its syntax, and how to use switch-case statement in programs with the help of examples.
Switch Statement
Switch statement consists of a variable or expression, and one more case blocks. Each case block has a value. The variable of expression is evaluated and matched against the case block values from top to bottom. If there is a match, respective case block executes.
Syntax
The syntax of switch statement is
switch (x) { case value1: //write your code here for x==value1 break; case value2: //write your code here for x==value2 break; case value3: //write your code here for x==value3 break; default: //default block }
where
switch
,case
,break
,default
are keywords.x
is variable or expression.value1
,value2
,value3
, .. are the values.
Examples
1. Print category of given item
In the following program, we print the category of the item using switch statement.
Based on the value in variable category
, respective case block executes.
PHP Program
<?php $category = 'lentil'; switch ($category) { case 'fruit': echo 'Its a fruit.'; break; case 'vegetable': echo 'Its a vegetable.'; break; case 'lentil': echo 'Its a lentil.'; break; case 'dairy': echo 'Its a dairy product.'; break; default: echo 'No category found.'; break; } ?>
Output
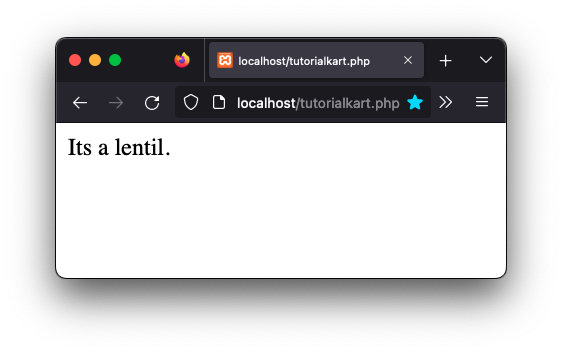
Conclusion
In this PHP Tutorial, we learned about switch statement, and how to use switch statement in a PHP program, with the help of examples.