In this tutorial, you shall be introduced to Strings in PHP, how to define string literals, how to print string values, how to create strings from different using characters, or numeric sequences, etc., with examples.
PHP Strings
In PHP, String is a sequence of characters.
Each character in a string is of size byte. Hence, PHP supports only 256-character set.
You can specify a string using single or double quotes. But each of these have their own meaning in interpreting some of the escaped characters. We will learn in detail.
Single Quoted Strings
To define a string in PHP, you can write the sequence of characters enclosed in single quotes.
Following is an example where we initialized a variable with a string using single quotes.
<?php
$string = 'Hello World!';
echo $string;
?>
Single quoted string does not interpret escaped sequences like \n, \r, \t, etc. But there is only one exception that you can escape a single quote. So, any backslash character that comes in a single quoted string is considered as just another character, except for the backslash followed by a single quote.
In the following program, we have demonstrated this behavior.
PHP Program
<?php
$string1 = 'Hello\' World!';
echo $string1;
echo '<br>';
$string2 = 'Hello\n\t World!';
echo $string2;
?>
Output
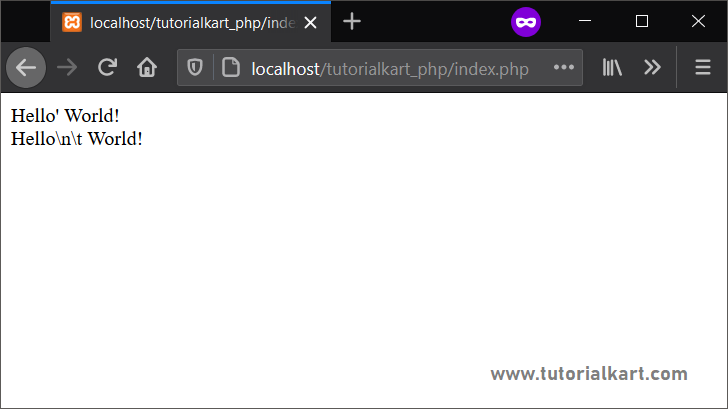
Double Quoted Strings
To define a string in PHP, you can write the sequence of characters enclosed in double quotes.
Following is an example where we initialized a variable with a string using double quotes.
<?php
$string = "Hello World!";
echo $string;
?>
Unlike single quoted strings, double quoted strings interpret escaped sequences like \n, \r, \t, etc.
Following table lists the escaped character by double quotes string, along with their description.
Escape Sequence | Meaning |
\n | New line |
\r | Carriage return |
\t | Horizontal tab |
\v | Vertical tab |
\e | Escape |
\f | Form feed |
\\ | Backslash |
\$ | Dollar sign |
\” | Double Quote |
In the following program, we have demonstrated how double quoted string escapes these special sequences.
PHP Program
<?php
$string = "Hello\n World!";
echo $string, '<br>';
$string = "Hello\r World!";
echo $string, '<br>';
$string = "Hello\t World!";
echo $string, '<br>';
$string = "Hello\v World!";
echo $string, '<br>';
$string = "Hello\e World!";
echo $string, '<br>';
$string = "Hello\f World!";
echo $string, '<br>';
$string = "Hello\\ World!";
echo $string, '<br>';
$string = "Hello\$ World!";
echo $string, '<br>';
$string = "Hello\" World!";
echo $string, '<br>';
?>
Output
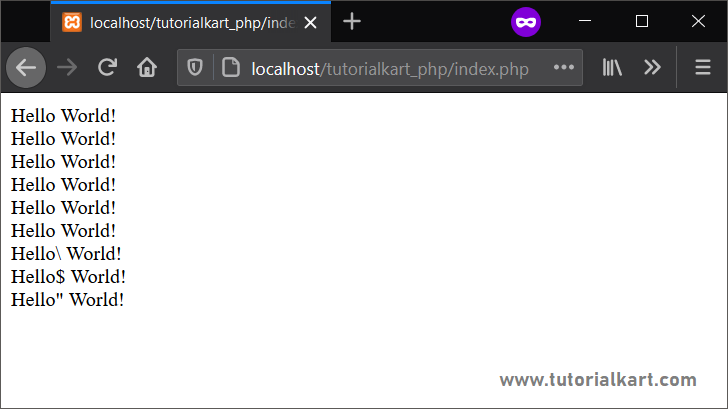
Octal Number Sequence
In a double quoted PHP String, you can specify a octal number using backslash. The octal number would be converted to a single byte. Anything that is overflow the byte is gone.
The sequence to write an octal number is
\[0-7]{1,3}
In the following program, we will write a string with octal numbers.
PHP Program
<?php
$string = "\110\145\154\154\157\127\157\162\154\144";
echo $string;
?>
Output
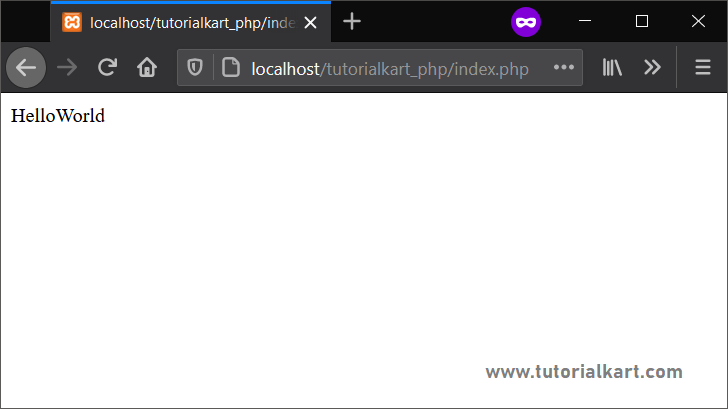
Hexadecimal Number Sequence
In a double quoted PHP String, you can specify a hexadecimal number using backslash. The hexadecimal number would be converted to a single byte. Anything that is overflow the byte is gone.
The sequence to write a hexadecimal number is
\x[0-9A-Fa-f]{1,2}
In the following program, we will write a string with hexadecimal numbers.
PHP Program
<?php
$string = "\x48\x65\x6c\x6c\x6f\x20\x57\x6f\x72\x6c\x64";
?>
Output
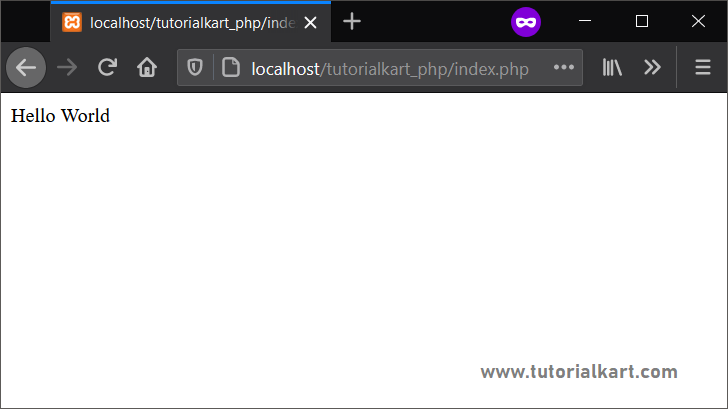
Conclusion
In this PHP Tutorial, we learned what Strings in PHP are, how to define a string literal, different ways to write a string, and their importance.