In this PHP tutorial, you shall learn to find the array length, the number of elements in one-dimensional or multi-dimensional arrays, using count() function, with examples.
PHP Array Length
To find the length of an array in PHP, use count() function. The count() function takes array as an argument, finds and returns the number of elements in the array.
Syntax of count()
The syntax of count() function to find array length is
count ( $arr [, int $mode = COUNT_NORMAL ] )
where
Parameter | Description |
array | Required. Specifies the array |
mode | Optional. Specifies the mode. Possible values: |
Function Return Value
count() function returns an integer representing number of elements in the array.
You will mostly use the following form of count() function.
count ( $arr )
We can store the returned value in a variable as shown below.
$length = count ( $arr )
If you pass multidimensional array, count($arr) returns length of array only in the first dimension.
If you want to count all elements in a multidimensional array recursively, pass COUNT_RECURSIVE as second argument, as shown in the following code sample.
count ( $arr, COUNT_RECURSIVE )
We will look into examples for each of these scenarios.
Examples
1. Find length of Indexed Array
This is a simple example to demonstrate the usage of count() function with array.
In the following program, we are initializing a PHP array with three numbers as elements. Then we are using count() function to find the number of elements in the array.
PHP Program
<?php
$arr = array( 41, 87, 66 );
echo count($arr);
?>
Output
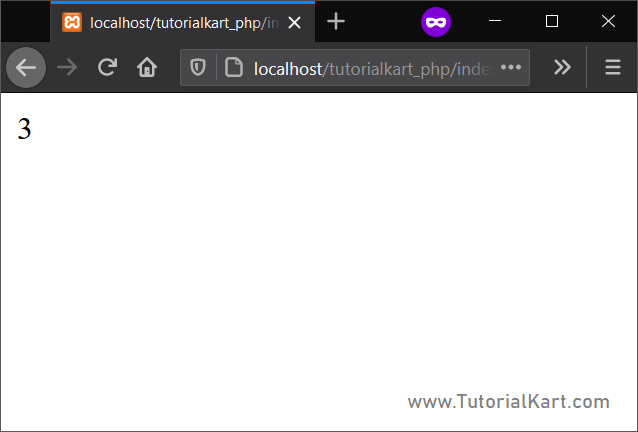
2. Find length of Associative Array
In the following program, we are initializing a PHP array with three key-value pairs. Then we are using count() function to find the number of elements in the array.
PHP Program
<?php
$arr = array(
"a" => 25,
"b" => 87,
"c" => 63
);
echo count($arr);
?>
Output
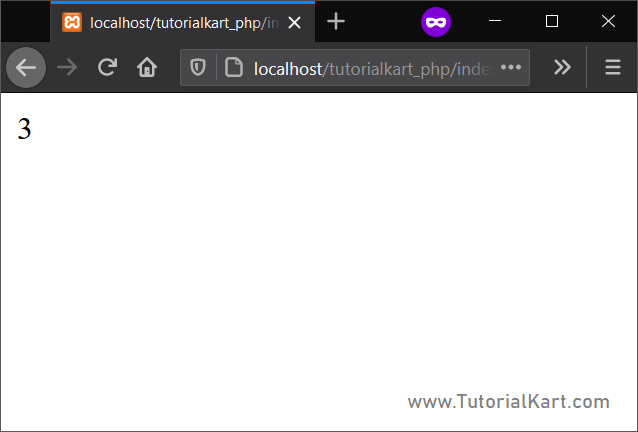
3. Find length of Multidimensional Array
In the following program, we are initializing a two dimensional PHP array. Then we are using count() function to find the number of elements in the array. Pass array as first argument and COUNT_RECURSIVE as second argument.
PHP Program
<?php
$arr = array(
"a" => array(41, 53, 9),
"b" => array(2, 96, 84, 16),
"c" => array(85, 7)
);
echo count($arr, COUNT_RECURSIVE);
?>
Output
There are three elements in the first dimension. And in the second dimension we have 3, 4 and 2 respectively. So, the total would be 3+3+4+2 = 12.
Run the above PHP program, and you get the following output in browser.
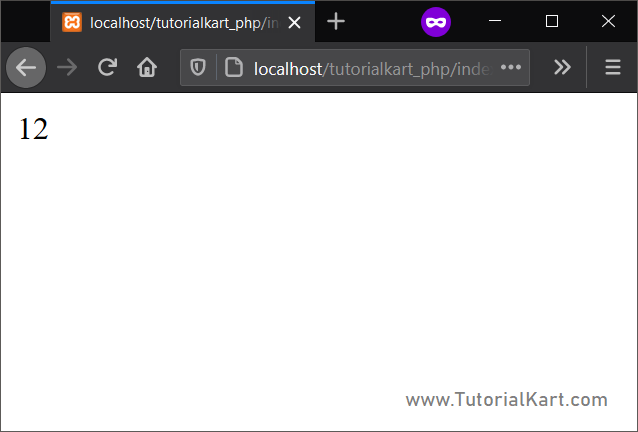
Note: The default value for second argument to count() function is COUNT_NORMAL.
So, if you do not pass any second argument to count() function, count() will return number of elements only in the first dimension of the array you passed.
In the following example, we have passed a two dimensional array, and no second argument to count().
PHP Program
<?php
$arr = array(
"a" => array(41, 53, 9),
"b" => array(2, 96, 84, 16),
"c" => array(85, 7)
);
echo count($arr);
?>
Program Output
The program will echo 3 as output, since there are only three element in the first dimension. The elements are with the keys “a”, “b” and “c”.
Conclusion
In this PHP Tutorial, we learned how to use count() function to find the length of given array.