In this PHP tutorial, you shall learn how to find the index of a specific substring in a given string using strpos() function, with example programs.
PHP – Find index of a substring in a string
To find the index of a substring in a string in PHP, call strpos()
function and pass given string and substring as arguments.
The strpos()
function returns an integer value of the index if the substring is present in the string, else, the function returns a boolean value of false.
Syntax
The syntax to find the index of substring search
in the string str
is
strpos($str, $search)
Examples
1. Positive Scenario – String contains search string
In this example, we take string values in str
and search
. We find the index of first occurrence of the search string search
in the string str
.
For given input values, strpos()
returns an integer value of 9
.
PHP Program
<?php
$str = 'Apple is red in color.';
$search = 'red';
$index = strpos($str, $search);
echo "Index of substring : " . $index;
?>
Output
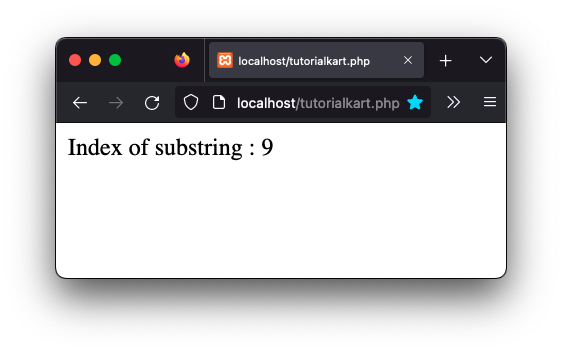
2. Negative Scenario – String does not contain search string
In this example, we take a string in str
and search string search
, such that the string str
does not contain search
.
For given input values, strpos()
returns false
.
PHP Program
<?php
$str = 'Apple is red in color.';
$search = 'yellow';
$index = strpos($str, $search);
echo "Index of substring : " . $index;
?>
Output
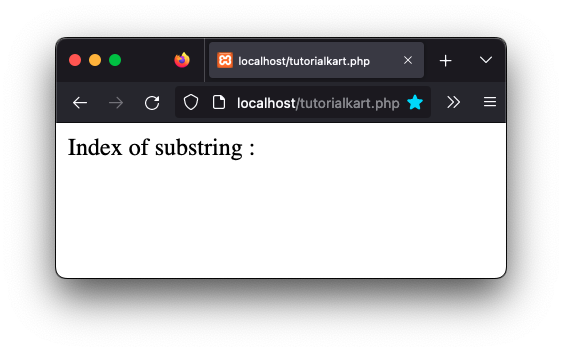
Conclusion
In this PHP Tutorial, we learned how to find the index of a substring (search string) in a given string, using strpos()
function, with the help of examples.