In this PHP tutorial, you shall learn about Associative Arrays, how to created associative arrays, access elements of associative arrays, and modify elements of associative arrays, with example programs.
PHP – Associative arrays
Associative Arrays are arrays in which the values are associated with keys. The keys can be used to access or modify the elements of array.
Create Associative array
To create an associative array in PHP, use array() function with the comma separated key-value pairs passed as argument to the function.
The syntax to create associative array using array() function is
$myarray = array(key=>value, key=>value, key=>value)
In the above code snippet, array() function returns an associative array.
Let us write a PHP program, where we shall create an associative array using array() function.
PHP Program
<?php $myArray = ["apple"=>52, "banana"=>84, "orange"=>12, "mango"=>13, "guava"=>25]; ?>
Access elements of Associative array
In the following example, we will create an associative array, and access a value using key.
PHP Program
<?php //associative array $myArray = ["apple"=>52, "banana"=>84, "orange"=>12, "mango"=>13, "guava"=>25];//modify element using index //access value $value = $myArray["banana"]; echo $value; ?>
Output
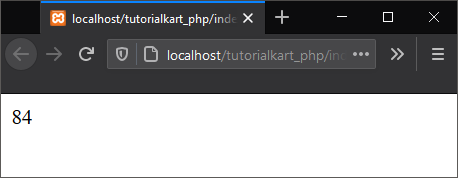
The value corresponding to the key “banana” is 84 in the array.
Modify elements of Associative array
To modify the elements of array using key, assign a new value to the array variable followed by the specific key in square brackets.
In the following example, we will initialize an associative array, and update the element with key “banana”.
PHP Program
<?php //associative array $myArray = ["apple"=>52, "banana"=>84, "orange"=>12, "mango"=>13, "guava"=>25];//modify element using index //access value $myArray["banana"] = 99; //print array foreach ($myArray as $key => $value) { echo $key . " - " . $value . "<br>"; } ?>
Output
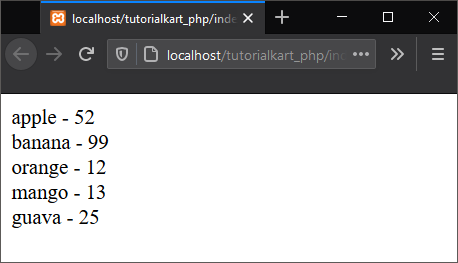
Conclusion
In this PHP Tutorial, we learned about associative arrays in PHP, how to use keys to access of modify elements of Associative Arrays, with the help of example PHP programs.