In this tutorial, you shall learn how to create an associative array from given two indexed arrays using array_combine() function, with syntax and examples.
PHP – Create an Associative Array from Two Indexed Array
To create an associative array from given two indexed arrays, where one array is used for keys and the other array is used for values, we can use array_combine()
function.
PHP array_combine()
function takes keys from one input array, takes values from another input array, combines these keys and values to form a new associative array, and returns this array.
In this tutorial, we will learn how to use array_combine()
function to combine array of keys and array of values to form a new array.
Syntax
The syntax of array_combine() function is
array_combine ( array $keys , array $values ) : array
where
Parameter | Description |
---|---|
keys | The array of keys used to create array. |
values | The array of values used to create array. |
Return Value
The array_combine() function returns an array formed by combining keys array and values array.
Warnings
The number of elements in keys and values arrays should match. If not, array_combine() raises a Warning.
Examples
1. Create Associative Array using Keys and Values
In this example, we will take two indexed arrays: first one is for keys, and the second one is for values. We will pass these arrays as arguments to array_combine()
function and print the resulting array.
PHP Program
<?php
$keys = array(65, 66, 67);
$values = array('A', 'B', 'C');
$result = array_combine($keys, $values);
print_r($result)
?>
Output
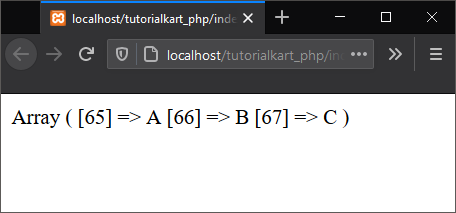
There are two observations that we can draw from this output. They are
- A key is picked from the keys array, and a value is picked from values array to create a key-value pair in the resulting array.
- The resulting array is an associative array.
2. Negative Scenario – Warning: array_combine(): Both parameters should have an equal number of elements
In this example, we will take keys array of length four and values array of length 3. We shall pass these arrays to array_combine() function. As the two parameters (arrays) do not have same lengths, array_combine() raises a Warning.
PHP Program
<?php
$keys = array(65, 66, 67, 68);
$values = array('A', 'B', 'C');
$result = array_combine($keys, $values);
print_r($result)
?>
Output
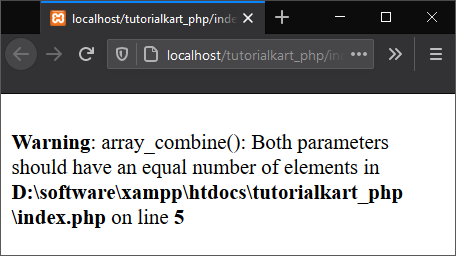
array_combine()
raises a warning that both parameters should have an equal number of elements.
Conclusion
In this PHP Tutorial, we learned how to create an associative array from two arrays: keys and values, using PHP Array array_combine() function.