In this PHP tutorial, you shall learn how to check if given string contains only lowercase letters using ctype_lower() function, with example programs.
PHP – Check if string contains only lowercase letters
To check if a string contains only lowercase letters in PHP, call ctype_lower()
function and pass given string as argument.
The ctype_lower()
function returns a boolean value representing if the string contains only lowercase characters.
Syntax
The syntax to check if string str
contains only lowercase letters is
ctype_lower($str)
The above expression returns a boolean value of true
if the string $str
contains only lowercase characters, or false
otherwise.
Examples
1. Positive Scenario – String contains only lowercase
In this example, we take a string in str
such that it contains only lowercase characters. For the given value of string, ctype_lower()
returns true
and if-block executes.
PHP Program
<?php $str = 'apple'; if ( ctype_lower($str) ) { echo 'string contains only lowercase'; } else { echo 'string does not contain only lowercase'; } ?>
Output
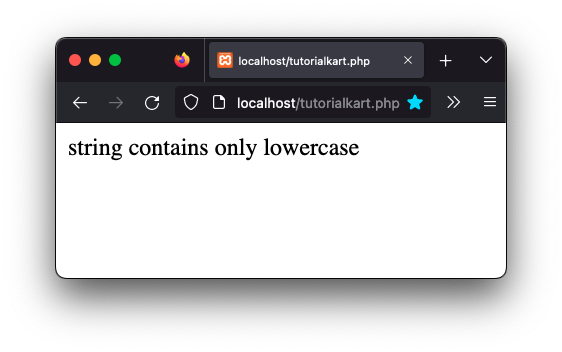
2. Negative Scenario – String contains uppercase also
In this example, we take a string in str
such that it contains some uppercase letters along with lowercase letters. For the given value of string, ctype_lower()
returns false
and else-block executes.
PHP Program
<?php $str = 'Apple'; if ( ctype_lower($str) ) { echo 'string contains only lowercase'; } else { echo 'string does not contain only lowercase'; } ?>
Output
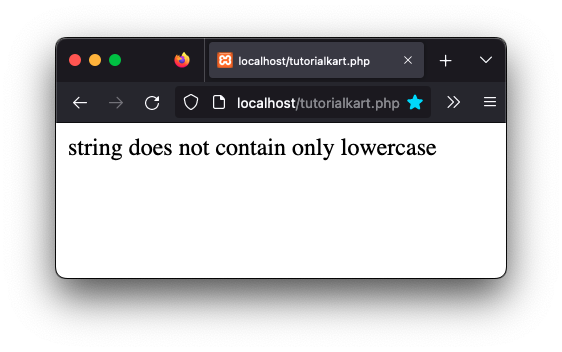
3. Negative Scenario – String contains digits also
In this example, we take a string in str
such that it contains some digits along with lowercase letters. For the given value of string, ctype_lower()
returns false
and else-block executes.
PHP Program
<?php $str = 'apple123'; if ( ctype_lower($str) ) { echo 'string contains only lowercase'; } else { echo 'string does not contain only lowercase'; } ?>
Output
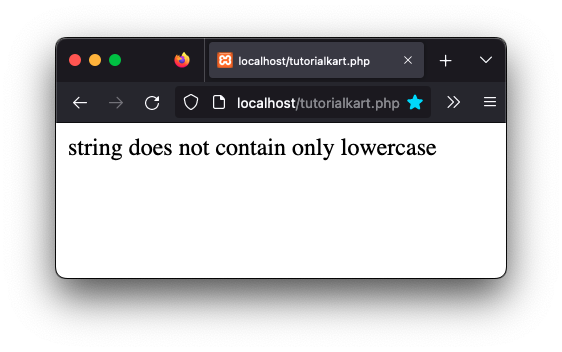
Conclusion
In this PHP Tutorial, we learned how to check if given string contains only lowercase characters, using ctype_lower()
function, with the help of examples.